Configure Spring for CORS
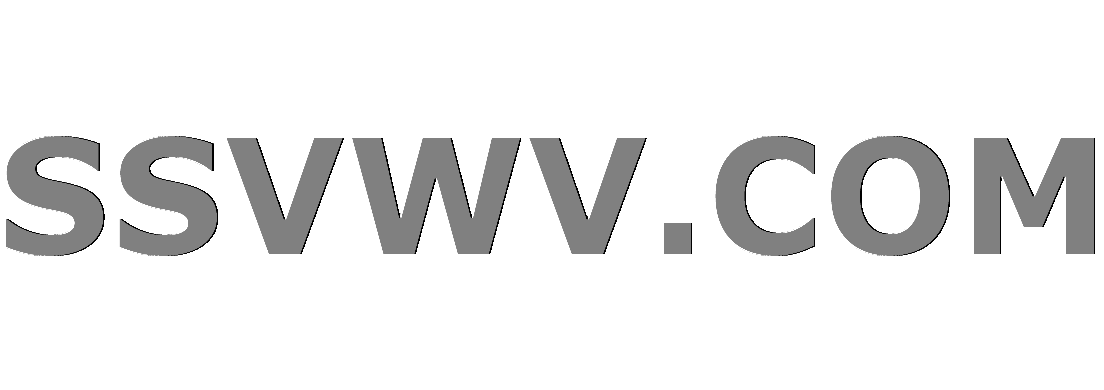
Multi tool use
I'm trying to configure Spring for CORS in order to use Angular web UI:
I tried this:
@Configuration
@ComponentScan("org.datalis.admin.config")
public class AppConfig {
@Bean
public static PropertySourcesPlaceholderConfigurer propertyConfigurer() {
PropertySourcesPlaceholderConfigurer conf = new PropertySourcesPlaceholderConfigurer();
conf.setLocation(new ClassPathResource("application.properties"));
return conf;
}
@Bean
public FilterRegistrationBean<CorsFilter> corsFilter() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
CorsConfiguration config = new CorsConfiguration();
config.setAllowCredentials(true);
config.addAllowedOrigin("127.0.0.1");
config.addAllowedHeader("*");
config.addAllowedMethod("*");
source.registerCorsConfiguration("/**", config);
FilterRegistrationBean<CorsFilter> bean = new FilterRegistrationBean<CorsFilter>(new CorsFilter(source));
bean.setOrder(0);
return bean;
}
}
Apache server with Angular FE is running with Wildly server on the same server so I configured 127.0.0.1 for source.
But still I get:
Access to XMLHttpRequest at 'http://123.123.123.123:8080/api/oauth/token' from origin 'http://123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
auth:1 Failed to load resource: the server responded with a status of 404 (Not Found)
Do you know how I can fix this issue?
Second way that I tried:
@Configuration
@EnableResourceServer
public class ResourceSecurityConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(ResourceServerSecurityConfigurer resources) {
resources.resourceId("resource_id").stateless(true);
}
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/users/**").permitAll()
.anyRequest().authenticated()
.and()
.cors().disable()
.authorizeRequests()
.antMatchers(HttpMethod.OPTIONS, "/**").permitAll()
.anyRequest()
.fullyAuthenticated()
.and()
.httpBasic()
.and()
.csrf().disable();
}
@Bean
public CorsConfigurationSource corsConfigurationSources() {
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("*"));
configuration.setAllowedMethods(Arrays.asList("GET", "POST", "PUT", "PATCH", "DELETE", "OPTIONS"));
configuration.setAllowedHeaders(Arrays.asList("authorization", "content-type", "x-auth-token"));
configuration.setExposedHeaders(Arrays.asList("x-auth-token"));
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
source.registerCorsConfiguration("/**", configuration);
return source;
}
}
With the second configuration I get has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
auth:1 Failed to load resource: the server responded with a status of 404 (Not Found)
What is the best way to achieve this result?
java angular spring spring-boot cors
This question has an open bounty worth +50
reputation from user1285928 ending in 5 days.
Looking for an answer drawing from credible and/or official sources.
add a comment |
I'm trying to configure Spring for CORS in order to use Angular web UI:
I tried this:
@Configuration
@ComponentScan("org.datalis.admin.config")
public class AppConfig {
@Bean
public static PropertySourcesPlaceholderConfigurer propertyConfigurer() {
PropertySourcesPlaceholderConfigurer conf = new PropertySourcesPlaceholderConfigurer();
conf.setLocation(new ClassPathResource("application.properties"));
return conf;
}
@Bean
public FilterRegistrationBean<CorsFilter> corsFilter() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
CorsConfiguration config = new CorsConfiguration();
config.setAllowCredentials(true);
config.addAllowedOrigin("127.0.0.1");
config.addAllowedHeader("*");
config.addAllowedMethod("*");
source.registerCorsConfiguration("/**", config);
FilterRegistrationBean<CorsFilter> bean = new FilterRegistrationBean<CorsFilter>(new CorsFilter(source));
bean.setOrder(0);
return bean;
}
}
Apache server with Angular FE is running with Wildly server on the same server so I configured 127.0.0.1 for source.
But still I get:
Access to XMLHttpRequest at 'http://123.123.123.123:8080/api/oauth/token' from origin 'http://123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
auth:1 Failed to load resource: the server responded with a status of 404 (Not Found)
Do you know how I can fix this issue?
Second way that I tried:
@Configuration
@EnableResourceServer
public class ResourceSecurityConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(ResourceServerSecurityConfigurer resources) {
resources.resourceId("resource_id").stateless(true);
}
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/users/**").permitAll()
.anyRequest().authenticated()
.and()
.cors().disable()
.authorizeRequests()
.antMatchers(HttpMethod.OPTIONS, "/**").permitAll()
.anyRequest()
.fullyAuthenticated()
.and()
.httpBasic()
.and()
.csrf().disable();
}
@Bean
public CorsConfigurationSource corsConfigurationSources() {
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("*"));
configuration.setAllowedMethods(Arrays.asList("GET", "POST", "PUT", "PATCH", "DELETE", "OPTIONS"));
configuration.setAllowedHeaders(Arrays.asList("authorization", "content-type", "x-auth-token"));
configuration.setExposedHeaders(Arrays.asList("x-auth-token"));
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
source.registerCorsConfiguration("/**", configuration);
return source;
}
}
With the second configuration I get has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
auth:1 Failed to load resource: the server responded with a status of 404 (Not Found)
What is the best way to achieve this result?
java angular spring spring-boot cors
This question has an open bounty worth +50
reputation from user1285928 ending in 5 days.
Looking for an answer drawing from credible and/or official sources.
Just as a side note, you might want to consider setting the precedence tobean.setOrder(Ordered.HIGHEST_PRECEDENCE)
instead of zero.
– Roddy of the Frozen Peas
Jan 18 at 16:33
Now I get No 'Access-Control-Allow-Origin' header is present on the requested resource.
– Peter Penzov
Jan 18 at 16:38
stackoverflow.com/a/53863603/10426557
– Jonathan Johx
Jan 18 at 19:18
@JonathanJohx I get Access to XMLHttpRequest at '123.123.123.123:8080/api/oauth/token' from origin '123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
– Peter Penzov
Jan 18 at 19:29
Any other proposals how to fix this?
– Peter Penzov
Jan 19 at 8:25
add a comment |
I'm trying to configure Spring for CORS in order to use Angular web UI:
I tried this:
@Configuration
@ComponentScan("org.datalis.admin.config")
public class AppConfig {
@Bean
public static PropertySourcesPlaceholderConfigurer propertyConfigurer() {
PropertySourcesPlaceholderConfigurer conf = new PropertySourcesPlaceholderConfigurer();
conf.setLocation(new ClassPathResource("application.properties"));
return conf;
}
@Bean
public FilterRegistrationBean<CorsFilter> corsFilter() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
CorsConfiguration config = new CorsConfiguration();
config.setAllowCredentials(true);
config.addAllowedOrigin("127.0.0.1");
config.addAllowedHeader("*");
config.addAllowedMethod("*");
source.registerCorsConfiguration("/**", config);
FilterRegistrationBean<CorsFilter> bean = new FilterRegistrationBean<CorsFilter>(new CorsFilter(source));
bean.setOrder(0);
return bean;
}
}
Apache server with Angular FE is running with Wildly server on the same server so I configured 127.0.0.1 for source.
But still I get:
Access to XMLHttpRequest at 'http://123.123.123.123:8080/api/oauth/token' from origin 'http://123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
auth:1 Failed to load resource: the server responded with a status of 404 (Not Found)
Do you know how I can fix this issue?
Second way that I tried:
@Configuration
@EnableResourceServer
public class ResourceSecurityConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(ResourceServerSecurityConfigurer resources) {
resources.resourceId("resource_id").stateless(true);
}
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/users/**").permitAll()
.anyRequest().authenticated()
.and()
.cors().disable()
.authorizeRequests()
.antMatchers(HttpMethod.OPTIONS, "/**").permitAll()
.anyRequest()
.fullyAuthenticated()
.and()
.httpBasic()
.and()
.csrf().disable();
}
@Bean
public CorsConfigurationSource corsConfigurationSources() {
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("*"));
configuration.setAllowedMethods(Arrays.asList("GET", "POST", "PUT", "PATCH", "DELETE", "OPTIONS"));
configuration.setAllowedHeaders(Arrays.asList("authorization", "content-type", "x-auth-token"));
configuration.setExposedHeaders(Arrays.asList("x-auth-token"));
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
source.registerCorsConfiguration("/**", configuration);
return source;
}
}
With the second configuration I get has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
auth:1 Failed to load resource: the server responded with a status of 404 (Not Found)
What is the best way to achieve this result?
java angular spring spring-boot cors
I'm trying to configure Spring for CORS in order to use Angular web UI:
I tried this:
@Configuration
@ComponentScan("org.datalis.admin.config")
public class AppConfig {
@Bean
public static PropertySourcesPlaceholderConfigurer propertyConfigurer() {
PropertySourcesPlaceholderConfigurer conf = new PropertySourcesPlaceholderConfigurer();
conf.setLocation(new ClassPathResource("application.properties"));
return conf;
}
@Bean
public FilterRegistrationBean<CorsFilter> corsFilter() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
CorsConfiguration config = new CorsConfiguration();
config.setAllowCredentials(true);
config.addAllowedOrigin("127.0.0.1");
config.addAllowedHeader("*");
config.addAllowedMethod("*");
source.registerCorsConfiguration("/**", config);
FilterRegistrationBean<CorsFilter> bean = new FilterRegistrationBean<CorsFilter>(new CorsFilter(source));
bean.setOrder(0);
return bean;
}
}
Apache server with Angular FE is running with Wildly server on the same server so I configured 127.0.0.1 for source.
But still I get:
Access to XMLHttpRequest at 'http://123.123.123.123:8080/api/oauth/token' from origin 'http://123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
auth:1 Failed to load resource: the server responded with a status of 404 (Not Found)
Do you know how I can fix this issue?
Second way that I tried:
@Configuration
@EnableResourceServer
public class ResourceSecurityConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(ResourceServerSecurityConfigurer resources) {
resources.resourceId("resource_id").stateless(true);
}
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/users/**").permitAll()
.anyRequest().authenticated()
.and()
.cors().disable()
.authorizeRequests()
.antMatchers(HttpMethod.OPTIONS, "/**").permitAll()
.anyRequest()
.fullyAuthenticated()
.and()
.httpBasic()
.and()
.csrf().disable();
}
@Bean
public CorsConfigurationSource corsConfigurationSources() {
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("*"));
configuration.setAllowedMethods(Arrays.asList("GET", "POST", "PUT", "PATCH", "DELETE", "OPTIONS"));
configuration.setAllowedHeaders(Arrays.asList("authorization", "content-type", "x-auth-token"));
configuration.setExposedHeaders(Arrays.asList("x-auth-token"));
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
source.registerCorsConfiguration("/**", configuration);
return source;
}
}
With the second configuration I get has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
auth:1 Failed to load resource: the server responded with a status of 404 (Not Found)
What is the best way to achieve this result?
java angular spring spring-boot cors
java angular spring spring-boot cors
edited 2 days ago
Peter Penzov
asked Jan 18 at 14:25
Peter PenzovPeter Penzov
7858180387
7858180387
This question has an open bounty worth +50
reputation from user1285928 ending in 5 days.
Looking for an answer drawing from credible and/or official sources.
This question has an open bounty worth +50
reputation from user1285928 ending in 5 days.
Looking for an answer drawing from credible and/or official sources.
Just as a side note, you might want to consider setting the precedence tobean.setOrder(Ordered.HIGHEST_PRECEDENCE)
instead of zero.
– Roddy of the Frozen Peas
Jan 18 at 16:33
Now I get No 'Access-Control-Allow-Origin' header is present on the requested resource.
– Peter Penzov
Jan 18 at 16:38
stackoverflow.com/a/53863603/10426557
– Jonathan Johx
Jan 18 at 19:18
@JonathanJohx I get Access to XMLHttpRequest at '123.123.123.123:8080/api/oauth/token' from origin '123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
– Peter Penzov
Jan 18 at 19:29
Any other proposals how to fix this?
– Peter Penzov
Jan 19 at 8:25
add a comment |
Just as a side note, you might want to consider setting the precedence tobean.setOrder(Ordered.HIGHEST_PRECEDENCE)
instead of zero.
– Roddy of the Frozen Peas
Jan 18 at 16:33
Now I get No 'Access-Control-Allow-Origin' header is present on the requested resource.
– Peter Penzov
Jan 18 at 16:38
stackoverflow.com/a/53863603/10426557
– Jonathan Johx
Jan 18 at 19:18
@JonathanJohx I get Access to XMLHttpRequest at '123.123.123.123:8080/api/oauth/token' from origin '123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
– Peter Penzov
Jan 18 at 19:29
Any other proposals how to fix this?
– Peter Penzov
Jan 19 at 8:25
Just as a side note, you might want to consider setting the precedence to
bean.setOrder(Ordered.HIGHEST_PRECEDENCE)
instead of zero.– Roddy of the Frozen Peas
Jan 18 at 16:33
Just as a side note, you might want to consider setting the precedence to
bean.setOrder(Ordered.HIGHEST_PRECEDENCE)
instead of zero.– Roddy of the Frozen Peas
Jan 18 at 16:33
Now I get No 'Access-Control-Allow-Origin' header is present on the requested resource.
– Peter Penzov
Jan 18 at 16:38
Now I get No 'Access-Control-Allow-Origin' header is present on the requested resource.
– Peter Penzov
Jan 18 at 16:38
stackoverflow.com/a/53863603/10426557
– Jonathan Johx
Jan 18 at 19:18
stackoverflow.com/a/53863603/10426557
– Jonathan Johx
Jan 18 at 19:18
@JonathanJohx I get Access to XMLHttpRequest at '123.123.123.123:8080/api/oauth/token' from origin '123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
– Peter Penzov
Jan 18 at 19:29
@JonathanJohx I get Access to XMLHttpRequest at '123.123.123.123:8080/api/oauth/token' from origin '123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
– Peter Penzov
Jan 18 at 19:29
Any other proposals how to fix this?
– Peter Penzov
Jan 19 at 8:25
Any other proposals how to fix this?
– Peter Penzov
Jan 19 at 8:25
add a comment |
5 Answers
5
active
oldest
votes
Your allowed origin is 127.0.0.1 but your client side has the ip 123.123.123.123. Try to change this:
config.addAllowedOrigin("127.0.0.1");
To this:
config.addAllowedOrigin("123.123.123.123");
New contributor
Raphael Alves is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Hi Raphael! Welcome to StackOverflow. I editted your post to format the code -- for future reference, code blocks are formatted by indenting them with four spaces.
– Roddy of the Frozen Peas
Jan 18 at 16:34
It's not working.
– Peter Penzov
Jan 18 at 18:46
You can try to add on your client the header "with credentials: true". You can do it on angular adding a interceptor on the http client. See stackoverflow.com/questions/38615205/…
– Raphael Alves
Jan 18 at 21:19
add a comment |
You need to add
@CrossOrigin
annotation to your rest controller class
Unfortunately it's not working.
– Peter Penzov
Jan 18 at 18:46
add a comment |
You need to tell Spring Security
to use the CORS Configuration you created.
In my project I configured Spring Security
in this way:
@Override
protected void configure(HttpSecurity http) throws Exception
{
http
.authorizeRequests()
.antMatchers("/rest/protected/**")
.authenticated()
//Other spring sec configruation and then:
.and()
.cors()
.configurationSource(corsConfigurationSource())
}
Where corsConfigurationSource()
is:
@Bean
CorsConfigurationSource corsConfigurationSource() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
boolean abilitaCors = new Boolean(env.getProperty("templating.oauth.enable.cors"));
if( abilitaCors )
{
if( logger.isWarnEnabled() )
{
logger.warn("CORS ABILITATI! Si assume ambiente di sviluppo");
}
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("http://localhost:4200","http://localhost:8080", "http://localhost:8180"));
configuration.setAllowedMethods(Arrays.asList( RequestMethod.GET.name(),
RequestMethod.POST.name(),
RequestMethod.OPTIONS.name(),
RequestMethod.DELETE.name(),
RequestMethod.PUT.name()));
configuration.setExposedHeaders(Arrays.asList("x-auth-token", "x-requested-with", "x-xsrf-token"));
configuration.setAllowedHeaders(Arrays.asList("X-Auth-Token","x-auth-token", "x-requested-with", "x-xsrf-token"));
source.registerCorsConfiguration("/**", configuration);
}
return source;
}
I hope it's useful
Angelo
I get again Access to XMLHttpRequest at'http://123.123.123.123:8080/api/oauth/token' from origin 'http://123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
Any other ideas?
– Peter Penzov
yesterday
From what I see I understand that: you must configure cors source with the IP address 123.123.123.123 more over the AJAX. Did you add also the IP address? Did you modify the CorsConfigurationSource by adding the right IP addess? Put on TRACE spring security logs
– Angelo Immediata
yesterday
How I can enable Spring security TRACE logs?
– Peter Penzov
yesterday
You can see my configuration here: github.com/rcbandit111/Spring_test
– Peter Penzov
yesterday
In yourlogback.xml
file add a spring logger like this one<logger name="org.springframework.security" level="TRACE"></logger>
– Angelo Immediata
yesterday
|
show 13 more comments
I recommend you to use a WebMvcConfigurer, and in the addCorsMappings method set the CORS configuration.
Somethingo like this
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://localhost:9798")
.allowedMethods("POST", "GET")
//.allowedHeaders("header1", "header2", "header3")
//.exposedHeaders("header1", "header2")
.allowCredentials(true).maxAge(3600);
}
}
Here there is a link with a fully functional Spring with CORS project, just download and run it.
https://github.com/reos79/spring-cors
It has a html page (person.html) this page does nothing but call the service on the port (9797). So you need to load this project twice, once on port 9797 to load the service and the other on port (9798). Then on you browser you call the page person on the server localhost:9798 and it will call the service on localhost:9797, in the file application.properties I configured the port.
do you know do I need to add some configuration in Apache and Angular?
– Peter Penzov
yesterday
No, the configuration is managed by spring on the server
– reos
yesterday
Here is the Wildfly config file using your configuration: pastebin.com/TzXPsNwt
– Peter Penzov
yesterday
Did you remove the Bean public CorsConfigurationSource corsConfigurationSources() ? and Bean public FilterRegistrationBean<CorsFilter> corsFilter() ?
– reos
yesterday
Yes, I did. But I will try again with clean setup later.
– Peter Penzov
yesterday
|
show 7 more comments
This is my working @Configuration
class to handle CORS requests used only in dev environment.
@Configuration
//@Profile(PROFILE_DEV)
public class CorsConfiguration {
@Bean
public WebMvcConfigurer corsConfigurer() {
return new WebMvcConfigurer() {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*")
.allowedHeaders("*")
.allowedMethods("*");
}
};
}
}
You have also to configure Spring Security to ignore HttpMethod.OPTIONS
used by preflight request (as the exception you mentioned)
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true, securedEnabled = true)
public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
//...
@Override
public void configure(WebSecurity web) throws Exception {
web.ignoring()
//others if you need
.antMatchers(HttpMethod.OPTIONS, "/**");
}
@Override
public void configure(HttpSecurity http) throws Exception {
http
.csrf()
.disable()
.exceptionHandling()
.and()
.headers()
.frameOptions()
.disable()
.and()
.authorizeRequests()
.antMatchers("/api/register").permitAll()
.antMatchers("/api/activate").permitAll()
.antMatchers("/api/authenticate").permitAll()
.antMatchers("/api/**").authenticated();
}
}
Because when you use cors you have Simple Request and Preflighted Request that triggers an HttpMethod.OPTIONS
I use also Spring security. Is this going to work for Spring Security?
– Peter Penzov
yesterday
updated answer with spring security OPTIONS configuration
– ValerioMC
yesterday
I tried your proposal combined wit the proposal from @Angelo Immediata but still I get the same issue. Any additional idea how to solve it?
– Peter Penzov
yesterday
My solution doesn't need to add other stuff. Try to leave the code as i explained, just be sure@Configuration
classes are correctly loaded. Tell me if you still have exception
– ValerioMC
yesterday
I tested only your code. Unfortunately the issue is not fixed.
– Peter Penzov
yesterday
|
show 9 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54255950%2fconfigure-spring-for-cors%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your allowed origin is 127.0.0.1 but your client side has the ip 123.123.123.123. Try to change this:
config.addAllowedOrigin("127.0.0.1");
To this:
config.addAllowedOrigin("123.123.123.123");
New contributor
Raphael Alves is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Hi Raphael! Welcome to StackOverflow. I editted your post to format the code -- for future reference, code blocks are formatted by indenting them with four spaces.
– Roddy of the Frozen Peas
Jan 18 at 16:34
It's not working.
– Peter Penzov
Jan 18 at 18:46
You can try to add on your client the header "with credentials: true". You can do it on angular adding a interceptor on the http client. See stackoverflow.com/questions/38615205/…
– Raphael Alves
Jan 18 at 21:19
add a comment |
Your allowed origin is 127.0.0.1 but your client side has the ip 123.123.123.123. Try to change this:
config.addAllowedOrigin("127.0.0.1");
To this:
config.addAllowedOrigin("123.123.123.123");
New contributor
Raphael Alves is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Hi Raphael! Welcome to StackOverflow. I editted your post to format the code -- for future reference, code blocks are formatted by indenting them with four spaces.
– Roddy of the Frozen Peas
Jan 18 at 16:34
It's not working.
– Peter Penzov
Jan 18 at 18:46
You can try to add on your client the header "with credentials: true". You can do it on angular adding a interceptor on the http client. See stackoverflow.com/questions/38615205/…
– Raphael Alves
Jan 18 at 21:19
add a comment |
Your allowed origin is 127.0.0.1 but your client side has the ip 123.123.123.123. Try to change this:
config.addAllowedOrigin("127.0.0.1");
To this:
config.addAllowedOrigin("123.123.123.123");
New contributor
Raphael Alves is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Your allowed origin is 127.0.0.1 but your client side has the ip 123.123.123.123. Try to change this:
config.addAllowedOrigin("127.0.0.1");
To this:
config.addAllowedOrigin("123.123.123.123");
New contributor
Raphael Alves is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited Jan 18 at 16:34
Roddy of the Frozen Peas
7,09482759
7,09482759
New contributor
Raphael Alves is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered Jan 18 at 16:27


Raphael AlvesRaphael Alves
292
292
New contributor
Raphael Alves is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Raphael Alves is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Raphael Alves is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Hi Raphael! Welcome to StackOverflow. I editted your post to format the code -- for future reference, code blocks are formatted by indenting them with four spaces.
– Roddy of the Frozen Peas
Jan 18 at 16:34
It's not working.
– Peter Penzov
Jan 18 at 18:46
You can try to add on your client the header "with credentials: true". You can do it on angular adding a interceptor on the http client. See stackoverflow.com/questions/38615205/…
– Raphael Alves
Jan 18 at 21:19
add a comment |
Hi Raphael! Welcome to StackOverflow. I editted your post to format the code -- for future reference, code blocks are formatted by indenting them with four spaces.
– Roddy of the Frozen Peas
Jan 18 at 16:34
It's not working.
– Peter Penzov
Jan 18 at 18:46
You can try to add on your client the header "with credentials: true". You can do it on angular adding a interceptor on the http client. See stackoverflow.com/questions/38615205/…
– Raphael Alves
Jan 18 at 21:19
Hi Raphael! Welcome to StackOverflow. I editted your post to format the code -- for future reference, code blocks are formatted by indenting them with four spaces.
– Roddy of the Frozen Peas
Jan 18 at 16:34
Hi Raphael! Welcome to StackOverflow. I editted your post to format the code -- for future reference, code blocks are formatted by indenting them with four spaces.
– Roddy of the Frozen Peas
Jan 18 at 16:34
It's not working.
– Peter Penzov
Jan 18 at 18:46
It's not working.
– Peter Penzov
Jan 18 at 18:46
You can try to add on your client the header "with credentials: true". You can do it on angular adding a interceptor on the http client. See stackoverflow.com/questions/38615205/…
– Raphael Alves
Jan 18 at 21:19
You can try to add on your client the header "with credentials: true". You can do it on angular adding a interceptor on the http client. See stackoverflow.com/questions/38615205/…
– Raphael Alves
Jan 18 at 21:19
add a comment |
You need to add
@CrossOrigin
annotation to your rest controller class
Unfortunately it's not working.
– Peter Penzov
Jan 18 at 18:46
add a comment |
You need to add
@CrossOrigin
annotation to your rest controller class
Unfortunately it's not working.
– Peter Penzov
Jan 18 at 18:46
add a comment |
You need to add
@CrossOrigin
annotation to your rest controller class
You need to add
@CrossOrigin
annotation to your rest controller class
answered Jan 18 at 15:32


Sasikumar MurugesanSasikumar Murugesan
2,28263352
2,28263352
Unfortunately it's not working.
– Peter Penzov
Jan 18 at 18:46
add a comment |
Unfortunately it's not working.
– Peter Penzov
Jan 18 at 18:46
Unfortunately it's not working.
– Peter Penzov
Jan 18 at 18:46
Unfortunately it's not working.
– Peter Penzov
Jan 18 at 18:46
add a comment |
You need to tell Spring Security
to use the CORS Configuration you created.
In my project I configured Spring Security
in this way:
@Override
protected void configure(HttpSecurity http) throws Exception
{
http
.authorizeRequests()
.antMatchers("/rest/protected/**")
.authenticated()
//Other spring sec configruation and then:
.and()
.cors()
.configurationSource(corsConfigurationSource())
}
Where corsConfigurationSource()
is:
@Bean
CorsConfigurationSource corsConfigurationSource() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
boolean abilitaCors = new Boolean(env.getProperty("templating.oauth.enable.cors"));
if( abilitaCors )
{
if( logger.isWarnEnabled() )
{
logger.warn("CORS ABILITATI! Si assume ambiente di sviluppo");
}
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("http://localhost:4200","http://localhost:8080", "http://localhost:8180"));
configuration.setAllowedMethods(Arrays.asList( RequestMethod.GET.name(),
RequestMethod.POST.name(),
RequestMethod.OPTIONS.name(),
RequestMethod.DELETE.name(),
RequestMethod.PUT.name()));
configuration.setExposedHeaders(Arrays.asList("x-auth-token", "x-requested-with", "x-xsrf-token"));
configuration.setAllowedHeaders(Arrays.asList("X-Auth-Token","x-auth-token", "x-requested-with", "x-xsrf-token"));
source.registerCorsConfiguration("/**", configuration);
}
return source;
}
I hope it's useful
Angelo
I get again Access to XMLHttpRequest at'http://123.123.123.123:8080/api/oauth/token' from origin 'http://123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
Any other ideas?
– Peter Penzov
yesterday
From what I see I understand that: you must configure cors source with the IP address 123.123.123.123 more over the AJAX. Did you add also the IP address? Did you modify the CorsConfigurationSource by adding the right IP addess? Put on TRACE spring security logs
– Angelo Immediata
yesterday
How I can enable Spring security TRACE logs?
– Peter Penzov
yesterday
You can see my configuration here: github.com/rcbandit111/Spring_test
– Peter Penzov
yesterday
In yourlogback.xml
file add a spring logger like this one<logger name="org.springframework.security" level="TRACE"></logger>
– Angelo Immediata
yesterday
|
show 13 more comments
You need to tell Spring Security
to use the CORS Configuration you created.
In my project I configured Spring Security
in this way:
@Override
protected void configure(HttpSecurity http) throws Exception
{
http
.authorizeRequests()
.antMatchers("/rest/protected/**")
.authenticated()
//Other spring sec configruation and then:
.and()
.cors()
.configurationSource(corsConfigurationSource())
}
Where corsConfigurationSource()
is:
@Bean
CorsConfigurationSource corsConfigurationSource() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
boolean abilitaCors = new Boolean(env.getProperty("templating.oauth.enable.cors"));
if( abilitaCors )
{
if( logger.isWarnEnabled() )
{
logger.warn("CORS ABILITATI! Si assume ambiente di sviluppo");
}
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("http://localhost:4200","http://localhost:8080", "http://localhost:8180"));
configuration.setAllowedMethods(Arrays.asList( RequestMethod.GET.name(),
RequestMethod.POST.name(),
RequestMethod.OPTIONS.name(),
RequestMethod.DELETE.name(),
RequestMethod.PUT.name()));
configuration.setExposedHeaders(Arrays.asList("x-auth-token", "x-requested-with", "x-xsrf-token"));
configuration.setAllowedHeaders(Arrays.asList("X-Auth-Token","x-auth-token", "x-requested-with", "x-xsrf-token"));
source.registerCorsConfiguration("/**", configuration);
}
return source;
}
I hope it's useful
Angelo
I get again Access to XMLHttpRequest at'http://123.123.123.123:8080/api/oauth/token' from origin 'http://123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
Any other ideas?
– Peter Penzov
yesterday
From what I see I understand that: you must configure cors source with the IP address 123.123.123.123 more over the AJAX. Did you add also the IP address? Did you modify the CorsConfigurationSource by adding the right IP addess? Put on TRACE spring security logs
– Angelo Immediata
yesterday
How I can enable Spring security TRACE logs?
– Peter Penzov
yesterday
You can see my configuration here: github.com/rcbandit111/Spring_test
– Peter Penzov
yesterday
In yourlogback.xml
file add a spring logger like this one<logger name="org.springframework.security" level="TRACE"></logger>
– Angelo Immediata
yesterday
|
show 13 more comments
You need to tell Spring Security
to use the CORS Configuration you created.
In my project I configured Spring Security
in this way:
@Override
protected void configure(HttpSecurity http) throws Exception
{
http
.authorizeRequests()
.antMatchers("/rest/protected/**")
.authenticated()
//Other spring sec configruation and then:
.and()
.cors()
.configurationSource(corsConfigurationSource())
}
Where corsConfigurationSource()
is:
@Bean
CorsConfigurationSource corsConfigurationSource() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
boolean abilitaCors = new Boolean(env.getProperty("templating.oauth.enable.cors"));
if( abilitaCors )
{
if( logger.isWarnEnabled() )
{
logger.warn("CORS ABILITATI! Si assume ambiente di sviluppo");
}
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("http://localhost:4200","http://localhost:8080", "http://localhost:8180"));
configuration.setAllowedMethods(Arrays.asList( RequestMethod.GET.name(),
RequestMethod.POST.name(),
RequestMethod.OPTIONS.name(),
RequestMethod.DELETE.name(),
RequestMethod.PUT.name()));
configuration.setExposedHeaders(Arrays.asList("x-auth-token", "x-requested-with", "x-xsrf-token"));
configuration.setAllowedHeaders(Arrays.asList("X-Auth-Token","x-auth-token", "x-requested-with", "x-xsrf-token"));
source.registerCorsConfiguration("/**", configuration);
}
return source;
}
I hope it's useful
Angelo
You need to tell Spring Security
to use the CORS Configuration you created.
In my project I configured Spring Security
in this way:
@Override
protected void configure(HttpSecurity http) throws Exception
{
http
.authorizeRequests()
.antMatchers("/rest/protected/**")
.authenticated()
//Other spring sec configruation and then:
.and()
.cors()
.configurationSource(corsConfigurationSource())
}
Where corsConfigurationSource()
is:
@Bean
CorsConfigurationSource corsConfigurationSource() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
boolean abilitaCors = new Boolean(env.getProperty("templating.oauth.enable.cors"));
if( abilitaCors )
{
if( logger.isWarnEnabled() )
{
logger.warn("CORS ABILITATI! Si assume ambiente di sviluppo");
}
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("http://localhost:4200","http://localhost:8080", "http://localhost:8180"));
configuration.setAllowedMethods(Arrays.asList( RequestMethod.GET.name(),
RequestMethod.POST.name(),
RequestMethod.OPTIONS.name(),
RequestMethod.DELETE.name(),
RequestMethod.PUT.name()));
configuration.setExposedHeaders(Arrays.asList("x-auth-token", "x-requested-with", "x-xsrf-token"));
configuration.setAllowedHeaders(Arrays.asList("X-Auth-Token","x-auth-token", "x-requested-with", "x-xsrf-token"));
source.registerCorsConfiguration("/**", configuration);
}
return source;
}
I hope it's useful
Angelo
answered yesterday


Angelo ImmediataAngelo Immediata
4,15641637
4,15641637
I get again Access to XMLHttpRequest at'http://123.123.123.123:8080/api/oauth/token' from origin 'http://123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
Any other ideas?
– Peter Penzov
yesterday
From what I see I understand that: you must configure cors source with the IP address 123.123.123.123 more over the AJAX. Did you add also the IP address? Did you modify the CorsConfigurationSource by adding the right IP addess? Put on TRACE spring security logs
– Angelo Immediata
yesterday
How I can enable Spring security TRACE logs?
– Peter Penzov
yesterday
You can see my configuration here: github.com/rcbandit111/Spring_test
– Peter Penzov
yesterday
In yourlogback.xml
file add a spring logger like this one<logger name="org.springframework.security" level="TRACE"></logger>
– Angelo Immediata
yesterday
|
show 13 more comments
I get again Access to XMLHttpRequest at'http://123.123.123.123:8080/api/oauth/token' from origin 'http://123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
Any other ideas?
– Peter Penzov
yesterday
From what I see I understand that: you must configure cors source with the IP address 123.123.123.123 more over the AJAX. Did you add also the IP address? Did you modify the CorsConfigurationSource by adding the right IP addess? Put on TRACE spring security logs
– Angelo Immediata
yesterday
How I can enable Spring security TRACE logs?
– Peter Penzov
yesterday
You can see my configuration here: github.com/rcbandit111/Spring_test
– Peter Penzov
yesterday
In yourlogback.xml
file add a spring logger like this one<logger name="org.springframework.security" level="TRACE"></logger>
– Angelo Immediata
yesterday
I get again Access to XMLHttpRequest at
'http://123.123.123.123:8080/api/oauth/token' from origin 'http://123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
Any other ideas?– Peter Penzov
yesterday
I get again Access to XMLHttpRequest at
'http://123.123.123.123:8080/api/oauth/token' from origin 'http://123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
Any other ideas?– Peter Penzov
yesterday
From what I see I understand that: you must configure cors source with the IP address 123.123.123.123 more over the AJAX. Did you add also the IP address? Did you modify the CorsConfigurationSource by adding the right IP addess? Put on TRACE spring security logs
– Angelo Immediata
yesterday
From what I see I understand that: you must configure cors source with the IP address 123.123.123.123 more over the AJAX. Did you add also the IP address? Did you modify the CorsConfigurationSource by adding the right IP addess? Put on TRACE spring security logs
– Angelo Immediata
yesterday
How I can enable Spring security TRACE logs?
– Peter Penzov
yesterday
How I can enable Spring security TRACE logs?
– Peter Penzov
yesterday
You can see my configuration here: github.com/rcbandit111/Spring_test
– Peter Penzov
yesterday
You can see my configuration here: github.com/rcbandit111/Spring_test
– Peter Penzov
yesterday
In your
logback.xml
file add a spring logger like this one <logger name="org.springframework.security" level="TRACE"></logger>
– Angelo Immediata
yesterday
In your
logback.xml
file add a spring logger like this one <logger name="org.springframework.security" level="TRACE"></logger>
– Angelo Immediata
yesterday
|
show 13 more comments
I recommend you to use a WebMvcConfigurer, and in the addCorsMappings method set the CORS configuration.
Somethingo like this
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://localhost:9798")
.allowedMethods("POST", "GET")
//.allowedHeaders("header1", "header2", "header3")
//.exposedHeaders("header1", "header2")
.allowCredentials(true).maxAge(3600);
}
}
Here there is a link with a fully functional Spring with CORS project, just download and run it.
https://github.com/reos79/spring-cors
It has a html page (person.html) this page does nothing but call the service on the port (9797). So you need to load this project twice, once on port 9797 to load the service and the other on port (9798). Then on you browser you call the page person on the server localhost:9798 and it will call the service on localhost:9797, in the file application.properties I configured the port.
do you know do I need to add some configuration in Apache and Angular?
– Peter Penzov
yesterday
No, the configuration is managed by spring on the server
– reos
yesterday
Here is the Wildfly config file using your configuration: pastebin.com/TzXPsNwt
– Peter Penzov
yesterday
Did you remove the Bean public CorsConfigurationSource corsConfigurationSources() ? and Bean public FilterRegistrationBean<CorsFilter> corsFilter() ?
– reos
yesterday
Yes, I did. But I will try again with clean setup later.
– Peter Penzov
yesterday
|
show 7 more comments
I recommend you to use a WebMvcConfigurer, and in the addCorsMappings method set the CORS configuration.
Somethingo like this
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://localhost:9798")
.allowedMethods("POST", "GET")
//.allowedHeaders("header1", "header2", "header3")
//.exposedHeaders("header1", "header2")
.allowCredentials(true).maxAge(3600);
}
}
Here there is a link with a fully functional Spring with CORS project, just download and run it.
https://github.com/reos79/spring-cors
It has a html page (person.html) this page does nothing but call the service on the port (9797). So you need to load this project twice, once on port 9797 to load the service and the other on port (9798). Then on you browser you call the page person on the server localhost:9798 and it will call the service on localhost:9797, in the file application.properties I configured the port.
do you know do I need to add some configuration in Apache and Angular?
– Peter Penzov
yesterday
No, the configuration is managed by spring on the server
– reos
yesterday
Here is the Wildfly config file using your configuration: pastebin.com/TzXPsNwt
– Peter Penzov
yesterday
Did you remove the Bean public CorsConfigurationSource corsConfigurationSources() ? and Bean public FilterRegistrationBean<CorsFilter> corsFilter() ?
– reos
yesterday
Yes, I did. But I will try again with clean setup later.
– Peter Penzov
yesterday
|
show 7 more comments
I recommend you to use a WebMvcConfigurer, and in the addCorsMappings method set the CORS configuration.
Somethingo like this
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://localhost:9798")
.allowedMethods("POST", "GET")
//.allowedHeaders("header1", "header2", "header3")
//.exposedHeaders("header1", "header2")
.allowCredentials(true).maxAge(3600);
}
}
Here there is a link with a fully functional Spring with CORS project, just download and run it.
https://github.com/reos79/spring-cors
It has a html page (person.html) this page does nothing but call the service on the port (9797). So you need to load this project twice, once on port 9797 to load the service and the other on port (9798). Then on you browser you call the page person on the server localhost:9798 and it will call the service on localhost:9797, in the file application.properties I configured the port.
I recommend you to use a WebMvcConfigurer, and in the addCorsMappings method set the CORS configuration.
Somethingo like this
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://localhost:9798")
.allowedMethods("POST", "GET")
//.allowedHeaders("header1", "header2", "header3")
//.exposedHeaders("header1", "header2")
.allowCredentials(true).maxAge(3600);
}
}
Here there is a link with a fully functional Spring with CORS project, just download and run it.
https://github.com/reos79/spring-cors
It has a html page (person.html) this page does nothing but call the service on the port (9797). So you need to load this project twice, once on port 9797 to load the service and the other on port (9798). Then on you browser you call the page person on the server localhost:9798 and it will call the service on localhost:9797, in the file application.properties I configured the port.
answered yesterday


reosreos
4,98631430
4,98631430
do you know do I need to add some configuration in Apache and Angular?
– Peter Penzov
yesterday
No, the configuration is managed by spring on the server
– reos
yesterday
Here is the Wildfly config file using your configuration: pastebin.com/TzXPsNwt
– Peter Penzov
yesterday
Did you remove the Bean public CorsConfigurationSource corsConfigurationSources() ? and Bean public FilterRegistrationBean<CorsFilter> corsFilter() ?
– reos
yesterday
Yes, I did. But I will try again with clean setup later.
– Peter Penzov
yesterday
|
show 7 more comments
do you know do I need to add some configuration in Apache and Angular?
– Peter Penzov
yesterday
No, the configuration is managed by spring on the server
– reos
yesterday
Here is the Wildfly config file using your configuration: pastebin.com/TzXPsNwt
– Peter Penzov
yesterday
Did you remove the Bean public CorsConfigurationSource corsConfigurationSources() ? and Bean public FilterRegistrationBean<CorsFilter> corsFilter() ?
– reos
yesterday
Yes, I did. But I will try again with clean setup later.
– Peter Penzov
yesterday
do you know do I need to add some configuration in Apache and Angular?
– Peter Penzov
yesterday
do you know do I need to add some configuration in Apache and Angular?
– Peter Penzov
yesterday
No, the configuration is managed by spring on the server
– reos
yesterday
No, the configuration is managed by spring on the server
– reos
yesterday
Here is the Wildfly config file using your configuration: pastebin.com/TzXPsNwt
– Peter Penzov
yesterday
Here is the Wildfly config file using your configuration: pastebin.com/TzXPsNwt
– Peter Penzov
yesterday
Did you remove the Bean public CorsConfigurationSource corsConfigurationSources() ? and Bean public FilterRegistrationBean<CorsFilter> corsFilter() ?
– reos
yesterday
Did you remove the Bean public CorsConfigurationSource corsConfigurationSources() ? and Bean public FilterRegistrationBean<CorsFilter> corsFilter() ?
– reos
yesterday
Yes, I did. But I will try again with clean setup later.
– Peter Penzov
yesterday
Yes, I did. But I will try again with clean setup later.
– Peter Penzov
yesterday
|
show 7 more comments
This is my working @Configuration
class to handle CORS requests used only in dev environment.
@Configuration
//@Profile(PROFILE_DEV)
public class CorsConfiguration {
@Bean
public WebMvcConfigurer corsConfigurer() {
return new WebMvcConfigurer() {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*")
.allowedHeaders("*")
.allowedMethods("*");
}
};
}
}
You have also to configure Spring Security to ignore HttpMethod.OPTIONS
used by preflight request (as the exception you mentioned)
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true, securedEnabled = true)
public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
//...
@Override
public void configure(WebSecurity web) throws Exception {
web.ignoring()
//others if you need
.antMatchers(HttpMethod.OPTIONS, "/**");
}
@Override
public void configure(HttpSecurity http) throws Exception {
http
.csrf()
.disable()
.exceptionHandling()
.and()
.headers()
.frameOptions()
.disable()
.and()
.authorizeRequests()
.antMatchers("/api/register").permitAll()
.antMatchers("/api/activate").permitAll()
.antMatchers("/api/authenticate").permitAll()
.antMatchers("/api/**").authenticated();
}
}
Because when you use cors you have Simple Request and Preflighted Request that triggers an HttpMethod.OPTIONS
I use also Spring security. Is this going to work for Spring Security?
– Peter Penzov
yesterday
updated answer with spring security OPTIONS configuration
– ValerioMC
yesterday
I tried your proposal combined wit the proposal from @Angelo Immediata but still I get the same issue. Any additional idea how to solve it?
– Peter Penzov
yesterday
My solution doesn't need to add other stuff. Try to leave the code as i explained, just be sure@Configuration
classes are correctly loaded. Tell me if you still have exception
– ValerioMC
yesterday
I tested only your code. Unfortunately the issue is not fixed.
– Peter Penzov
yesterday
|
show 9 more comments
This is my working @Configuration
class to handle CORS requests used only in dev environment.
@Configuration
//@Profile(PROFILE_DEV)
public class CorsConfiguration {
@Bean
public WebMvcConfigurer corsConfigurer() {
return new WebMvcConfigurer() {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*")
.allowedHeaders("*")
.allowedMethods("*");
}
};
}
}
You have also to configure Spring Security to ignore HttpMethod.OPTIONS
used by preflight request (as the exception you mentioned)
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true, securedEnabled = true)
public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
//...
@Override
public void configure(WebSecurity web) throws Exception {
web.ignoring()
//others if you need
.antMatchers(HttpMethod.OPTIONS, "/**");
}
@Override
public void configure(HttpSecurity http) throws Exception {
http
.csrf()
.disable()
.exceptionHandling()
.and()
.headers()
.frameOptions()
.disable()
.and()
.authorizeRequests()
.antMatchers("/api/register").permitAll()
.antMatchers("/api/activate").permitAll()
.antMatchers("/api/authenticate").permitAll()
.antMatchers("/api/**").authenticated();
}
}
Because when you use cors you have Simple Request and Preflighted Request that triggers an HttpMethod.OPTIONS
I use also Spring security. Is this going to work for Spring Security?
– Peter Penzov
yesterday
updated answer with spring security OPTIONS configuration
– ValerioMC
yesterday
I tried your proposal combined wit the proposal from @Angelo Immediata but still I get the same issue. Any additional idea how to solve it?
– Peter Penzov
yesterday
My solution doesn't need to add other stuff. Try to leave the code as i explained, just be sure@Configuration
classes are correctly loaded. Tell me if you still have exception
– ValerioMC
yesterday
I tested only your code. Unfortunately the issue is not fixed.
– Peter Penzov
yesterday
|
show 9 more comments
This is my working @Configuration
class to handle CORS requests used only in dev environment.
@Configuration
//@Profile(PROFILE_DEV)
public class CorsConfiguration {
@Bean
public WebMvcConfigurer corsConfigurer() {
return new WebMvcConfigurer() {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*")
.allowedHeaders("*")
.allowedMethods("*");
}
};
}
}
You have also to configure Spring Security to ignore HttpMethod.OPTIONS
used by preflight request (as the exception you mentioned)
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true, securedEnabled = true)
public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
//...
@Override
public void configure(WebSecurity web) throws Exception {
web.ignoring()
//others if you need
.antMatchers(HttpMethod.OPTIONS, "/**");
}
@Override
public void configure(HttpSecurity http) throws Exception {
http
.csrf()
.disable()
.exceptionHandling()
.and()
.headers()
.frameOptions()
.disable()
.and()
.authorizeRequests()
.antMatchers("/api/register").permitAll()
.antMatchers("/api/activate").permitAll()
.antMatchers("/api/authenticate").permitAll()
.antMatchers("/api/**").authenticated();
}
}
Because when you use cors you have Simple Request and Preflighted Request that triggers an HttpMethod.OPTIONS
This is my working @Configuration
class to handle CORS requests used only in dev environment.
@Configuration
//@Profile(PROFILE_DEV)
public class CorsConfiguration {
@Bean
public WebMvcConfigurer corsConfigurer() {
return new WebMvcConfigurer() {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*")
.allowedHeaders("*")
.allowedMethods("*");
}
};
}
}
You have also to configure Spring Security to ignore HttpMethod.OPTIONS
used by preflight request (as the exception you mentioned)
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true, securedEnabled = true)
public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
//...
@Override
public void configure(WebSecurity web) throws Exception {
web.ignoring()
//others if you need
.antMatchers(HttpMethod.OPTIONS, "/**");
}
@Override
public void configure(HttpSecurity http) throws Exception {
http
.csrf()
.disable()
.exceptionHandling()
.and()
.headers()
.frameOptions()
.disable()
.and()
.authorizeRequests()
.antMatchers("/api/register").permitAll()
.antMatchers("/api/activate").permitAll()
.antMatchers("/api/authenticate").permitAll()
.antMatchers("/api/**").authenticated();
}
}
Because when you use cors you have Simple Request and Preflighted Request that triggers an HttpMethod.OPTIONS
edited yesterday
answered yesterday
ValerioMCValerioMC
87549
87549
I use also Spring security. Is this going to work for Spring Security?
– Peter Penzov
yesterday
updated answer with spring security OPTIONS configuration
– ValerioMC
yesterday
I tried your proposal combined wit the proposal from @Angelo Immediata but still I get the same issue. Any additional idea how to solve it?
– Peter Penzov
yesterday
My solution doesn't need to add other stuff. Try to leave the code as i explained, just be sure@Configuration
classes are correctly loaded. Tell me if you still have exception
– ValerioMC
yesterday
I tested only your code. Unfortunately the issue is not fixed.
– Peter Penzov
yesterday
|
show 9 more comments
I use also Spring security. Is this going to work for Spring Security?
– Peter Penzov
yesterday
updated answer with spring security OPTIONS configuration
– ValerioMC
yesterday
I tried your proposal combined wit the proposal from @Angelo Immediata but still I get the same issue. Any additional idea how to solve it?
– Peter Penzov
yesterday
My solution doesn't need to add other stuff. Try to leave the code as i explained, just be sure@Configuration
classes are correctly loaded. Tell me if you still have exception
– ValerioMC
yesterday
I tested only your code. Unfortunately the issue is not fixed.
– Peter Penzov
yesterday
I use also Spring security. Is this going to work for Spring Security?
– Peter Penzov
yesterday
I use also Spring security. Is this going to work for Spring Security?
– Peter Penzov
yesterday
updated answer with spring security OPTIONS configuration
– ValerioMC
yesterday
updated answer with spring security OPTIONS configuration
– ValerioMC
yesterday
I tried your proposal combined wit the proposal from @Angelo Immediata but still I get the same issue. Any additional idea how to solve it?
– Peter Penzov
yesterday
I tried your proposal combined wit the proposal from @Angelo Immediata but still I get the same issue. Any additional idea how to solve it?
– Peter Penzov
yesterday
My solution doesn't need to add other stuff. Try to leave the code as i explained, just be sure
@Configuration
classes are correctly loaded. Tell me if you still have exception– ValerioMC
yesterday
My solution doesn't need to add other stuff. Try to leave the code as i explained, just be sure
@Configuration
classes are correctly loaded. Tell me if you still have exception– ValerioMC
yesterday
I tested only your code. Unfortunately the issue is not fixed.
– Peter Penzov
yesterday
I tested only your code. Unfortunately the issue is not fixed.
– Peter Penzov
yesterday
|
show 9 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54255950%2fconfigure-spring-for-cors%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yUuzF9jbGp9PLDvZixziKSj gPWbeUCcZKD qvqt8P0EZDMopRNtmehJVEAWdcxt2fsEUR,NlV1ZUyZwjXhJhJStRM pLld sae q
Just as a side note, you might want to consider setting the precedence to
bean.setOrder(Ordered.HIGHEST_PRECEDENCE)
instead of zero.– Roddy of the Frozen Peas
Jan 18 at 16:33
Now I get No 'Access-Control-Allow-Origin' header is present on the requested resource.
– Peter Penzov
Jan 18 at 16:38
stackoverflow.com/a/53863603/10426557
– Jonathan Johx
Jan 18 at 19:18
@JonathanJohx I get Access to XMLHttpRequest at '123.123.123.123:8080/api/oauth/token' from origin '123.123.123.123' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: It does not have HTTP ok status.
– Peter Penzov
Jan 18 at 19:29
Any other proposals how to fix this?
– Peter Penzov
Jan 19 at 8:25