Splitting the list in to two parts in Scala
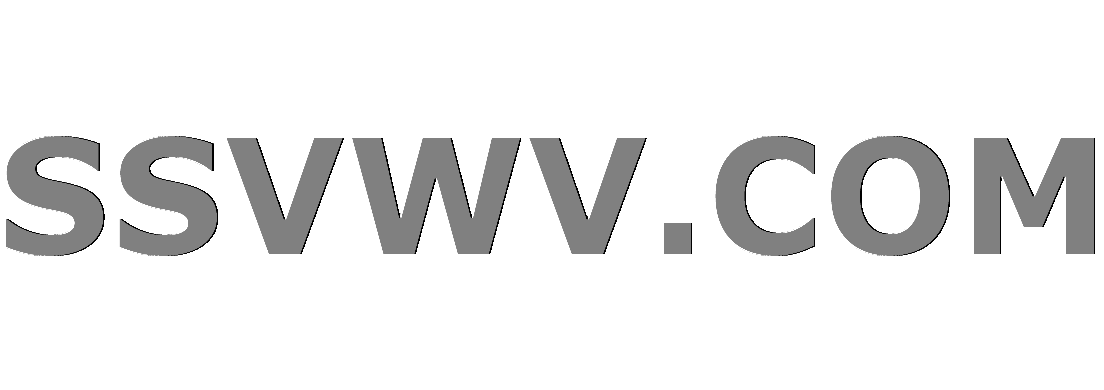
Multi tool use
I have a List with n as 3 ls as List(a,b,c,d,e) My query is to code for the (3,List(a,b,c,d,e)) I want to split them in to two parts such as List(a,b,c),List(d,e). For this the scala program is like below.
I don't understand val(pre,post). why it is used and what do we get from it? can someone please elaborate?
def splitRecursive[A](n: Int, ls: List[A]): (List[A], List[A]) = (n, ls) match {
case (_, Nil) => (Nil, Nil)
case (0, list) => (Nil, list)
case (n, h :: tail) => {
val (pre, post) = splitRecursive(n - 1, tail)
(h :: pre, post)
}
}
scala
add a comment |
I have a List with n as 3 ls as List(a,b,c,d,e) My query is to code for the (3,List(a,b,c,d,e)) I want to split them in to two parts such as List(a,b,c),List(d,e). For this the scala program is like below.
I don't understand val(pre,post). why it is used and what do we get from it? can someone please elaborate?
def splitRecursive[A](n: Int, ls: List[A]): (List[A], List[A]) = (n, ls) match {
case (_, Nil) => (Nil, Nil)
case (0, list) => (Nil, list)
case (n, h :: tail) => {
val (pre, post) = splitRecursive(n - 1, tail)
(h :: pre, post)
}
}
scala
add a comment |
I have a List with n as 3 ls as List(a,b,c,d,e) My query is to code for the (3,List(a,b,c,d,e)) I want to split them in to two parts such as List(a,b,c),List(d,e). For this the scala program is like below.
I don't understand val(pre,post). why it is used and what do we get from it? can someone please elaborate?
def splitRecursive[A](n: Int, ls: List[A]): (List[A], List[A]) = (n, ls) match {
case (_, Nil) => (Nil, Nil)
case (0, list) => (Nil, list)
case (n, h :: tail) => {
val (pre, post) = splitRecursive(n - 1, tail)
(h :: pre, post)
}
}
scala
I have a List with n as 3 ls as List(a,b,c,d,e) My query is to code for the (3,List(a,b,c,d,e)) I want to split them in to two parts such as List(a,b,c),List(d,e). For this the scala program is like below.
I don't understand val(pre,post). why it is used and what do we get from it? can someone please elaborate?
def splitRecursive[A](n: Int, ls: List[A]): (List[A], List[A]) = (n, ls) match {
case (_, Nil) => (Nil, Nil)
case (0, list) => (Nil, list)
case (n, h :: tail) => {
val (pre, post) = splitRecursive(n - 1, tail)
(h :: pre, post)
}
}
scala
scala
asked Jan 18 at 23:45
user2708013user2708013
215
215
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Your splitRecursive
function returns a pair of lists. To get the two lists out of the pair, you can either fetch them like this:
val result = splitRecursive(n - 1, tail)
val pre = result._1
val post = result._2
Or you can use destructuring to get them without first having to bind the pair to result
. That is what the syntax in splitRecursive
is doing.
val (pre, post) = splitRecursive(n - 1, tail)
It is simply a convenient way to get the elements out of a pair (or some other structure that can be destructured).
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54262811%2fsplitting-the-list-in-to-two-parts-in-scala%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your splitRecursive
function returns a pair of lists. To get the two lists out of the pair, you can either fetch them like this:
val result = splitRecursive(n - 1, tail)
val pre = result._1
val post = result._2
Or you can use destructuring to get them without first having to bind the pair to result
. That is what the syntax in splitRecursive
is doing.
val (pre, post) = splitRecursive(n - 1, tail)
It is simply a convenient way to get the elements out of a pair (or some other structure that can be destructured).
add a comment |
Your splitRecursive
function returns a pair of lists. To get the two lists out of the pair, you can either fetch them like this:
val result = splitRecursive(n - 1, tail)
val pre = result._1
val post = result._2
Or you can use destructuring to get them without first having to bind the pair to result
. That is what the syntax in splitRecursive
is doing.
val (pre, post) = splitRecursive(n - 1, tail)
It is simply a convenient way to get the elements out of a pair (or some other structure that can be destructured).
add a comment |
Your splitRecursive
function returns a pair of lists. To get the two lists out of the pair, you can either fetch them like this:
val result = splitRecursive(n - 1, tail)
val pre = result._1
val post = result._2
Or you can use destructuring to get them without first having to bind the pair to result
. That is what the syntax in splitRecursive
is doing.
val (pre, post) = splitRecursive(n - 1, tail)
It is simply a convenient way to get the elements out of a pair (or some other structure that can be destructured).
Your splitRecursive
function returns a pair of lists. To get the two lists out of the pair, you can either fetch them like this:
val result = splitRecursive(n - 1, tail)
val pre = result._1
val post = result._2
Or you can use destructuring to get them without first having to bind the pair to result
. That is what the syntax in splitRecursive
is doing.
val (pre, post) = splitRecursive(n - 1, tail)
It is simply a convenient way to get the elements out of a pair (or some other structure that can be destructured).
edited Jan 19 at 1:15
SergGr
20.9k22243
20.9k22243
answered Jan 18 at 23:58
marstranmarstran
10.1k12441
10.1k12441
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54262811%2fsplitting-the-list-in-to-two-parts-in-scala%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6M8,Kh2q oSnr10U1,y70YumgLK8T nHDn7 oc,GrFPpDqrnF,HkMjrhqKbcLrXMd