Yii2 include datetimepicker in ActiveForm
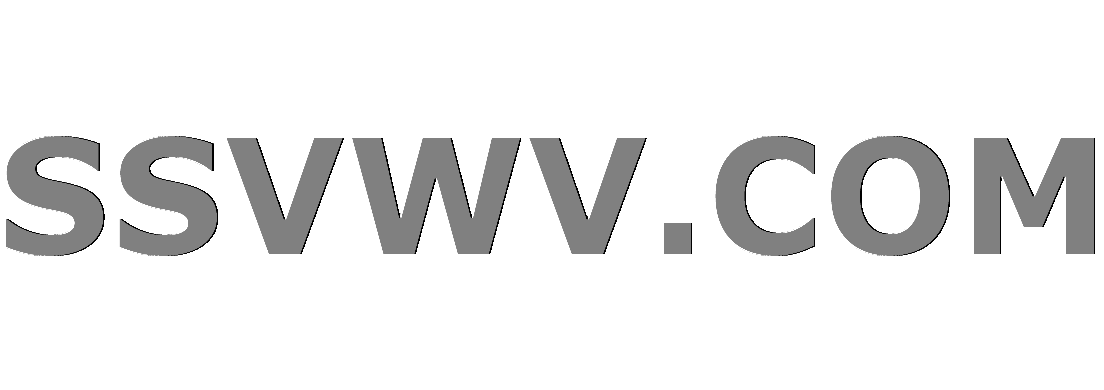
Multi tool use
I'm using Activeform and I need a custom field to insert datetime values.
I've found a possible solution myself with kartik DateTimePicker although it is much more complex than I wanted, anyway I don't know how to put the value in the model's attribute.
use kartikdatetimeDateTimePicker;
<?php $form = ActiveForm::begin(); ?>
// my working field using kartik library
<?= $form->field($model, 'course')->widget(kartikselect2Select2::className(),[
'data' => $data,
'language' => 'it',
'options' => ['placeholder' => 'Select a Course ...'],
'pluginOptions' => [
'allowClear' => true
],
]);?>
// the field I need to implement placing the result in $form->field($model, 'opening_date')
<?php //echo '<label style="position:absolute; top:5px; text-align:left;">Recording Time</label>';
echo '<label>Start Date/Time</label>';
echo DateTimePicker::widget([
'name' => 'opening_date',
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]);
?>
I accept also solutions offering a simpler way to show the field.
php yii2
add a comment |
I'm using Activeform and I need a custom field to insert datetime values.
I've found a possible solution myself with kartik DateTimePicker although it is much more complex than I wanted, anyway I don't know how to put the value in the model's attribute.
use kartikdatetimeDateTimePicker;
<?php $form = ActiveForm::begin(); ?>
// my working field using kartik library
<?= $form->field($model, 'course')->widget(kartikselect2Select2::className(),[
'data' => $data,
'language' => 'it',
'options' => ['placeholder' => 'Select a Course ...'],
'pluginOptions' => [
'allowClear' => true
],
]);?>
// the field I need to implement placing the result in $form->field($model, 'opening_date')
<?php //echo '<label style="position:absolute; top:5px; text-align:left;">Recording Time</label>';
echo '<label>Start Date/Time</label>';
echo DateTimePicker::widget([
'name' => 'opening_date',
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]);
?>
I accept also solutions offering a simpler way to show the field.
php yii2
ummmm... the question isnt clear you might need to provide more detail, why are you using a separate input field to submit the values when you are already using the widget, which also does the same?
– Muhammad Omer Aslam
2 days ago
add a comment |
I'm using Activeform and I need a custom field to insert datetime values.
I've found a possible solution myself with kartik DateTimePicker although it is much more complex than I wanted, anyway I don't know how to put the value in the model's attribute.
use kartikdatetimeDateTimePicker;
<?php $form = ActiveForm::begin(); ?>
// my working field using kartik library
<?= $form->field($model, 'course')->widget(kartikselect2Select2::className(),[
'data' => $data,
'language' => 'it',
'options' => ['placeholder' => 'Select a Course ...'],
'pluginOptions' => [
'allowClear' => true
],
]);?>
// the field I need to implement placing the result in $form->field($model, 'opening_date')
<?php //echo '<label style="position:absolute; top:5px; text-align:left;">Recording Time</label>';
echo '<label>Start Date/Time</label>';
echo DateTimePicker::widget([
'name' => 'opening_date',
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]);
?>
I accept also solutions offering a simpler way to show the field.
php yii2
I'm using Activeform and I need a custom field to insert datetime values.
I've found a possible solution myself with kartik DateTimePicker although it is much more complex than I wanted, anyway I don't know how to put the value in the model's attribute.
use kartikdatetimeDateTimePicker;
<?php $form = ActiveForm::begin(); ?>
// my working field using kartik library
<?= $form->field($model, 'course')->widget(kartikselect2Select2::className(),[
'data' => $data,
'language' => 'it',
'options' => ['placeholder' => 'Select a Course ...'],
'pluginOptions' => [
'allowClear' => true
],
]);?>
// the field I need to implement placing the result in $form->field($model, 'opening_date')
<?php //echo '<label style="position:absolute; top:5px; text-align:left;">Recording Time</label>';
echo '<label>Start Date/Time</label>';
echo DateTimePicker::widget([
'name' => 'opening_date',
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]);
?>
I accept also solutions offering a simpler way to show the field.
php yii2
php yii2
asked 2 days ago
gipsygipsy
245
245
ummmm... the question isnt clear you might need to provide more detail, why are you using a separate input field to submit the values when you are already using the widget, which also does the same?
– Muhammad Omer Aslam
2 days ago
add a comment |
ummmm... the question isnt clear you might need to provide more detail, why are you using a separate input field to submit the values when you are already using the widget, which also does the same?
– Muhammad Omer Aslam
2 days ago
ummmm... the question isnt clear you might need to provide more detail, why are you using a separate input field to submit the values when you are already using the widget, which also does the same?
– Muhammad Omer Aslam
2 days ago
ummmm... the question isnt clear you might need to provide more detail, why are you using a separate input field to submit the values when you are already using the widget, which also does the same?
– Muhammad Omer Aslam
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
If i understand correctly you are asking about using the widget with the model rather than calling it statically as you are currently copying the value to another ActiveForm field and then submitting it. If that is correct you can use the widget as a part of ActiveForm
field so that you don't have to insert the date into a separate model input to submit and save, see below.
<?php echo $form->field($model, 'opening_date')->widget(
DateTimePicker::class,
[
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]
);
?>
or you can pass the $model
and attribute
to your current code when calling the widget like below
echo DateTimePicker::widget(
[
'model' => $model,
'attribute' => 'opening_date',
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]
);
You can either pass the name
/model
& attribute
option see details
I tried with the first and this is the error: "Setting unknown property: yiijuiDatePicker::convertFormat"
– gipsy
2 days ago
@gipsy sorry my bad i usedDatePicker
instead ofDateTimePicker
i just updated it in the answer change it toDateTimePicker
and it will work
– Muhammad Omer Aslam
2 days ago
Thank you, now it works, it's just that the input is too complex, do you know other methods to create a model input field?
– gipsy
2 days ago
@gipsy what do you mean by input is too complex? and you can create with the above given methods when using a widget with input field , if its a normal field then you can also use theyiihelpersHtml
class too , for creating model inputs. do mark the answer as correct if it worked for you
– Muhammad Omer Aslam
2 days ago
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54252760%2fyii2-include-datetimepicker-in-activeform%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If i understand correctly you are asking about using the widget with the model rather than calling it statically as you are currently copying the value to another ActiveForm field and then submitting it. If that is correct you can use the widget as a part of ActiveForm
field so that you don't have to insert the date into a separate model input to submit and save, see below.
<?php echo $form->field($model, 'opening_date')->widget(
DateTimePicker::class,
[
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]
);
?>
or you can pass the $model
and attribute
to your current code when calling the widget like below
echo DateTimePicker::widget(
[
'model' => $model,
'attribute' => 'opening_date',
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]
);
You can either pass the name
/model
& attribute
option see details
I tried with the first and this is the error: "Setting unknown property: yiijuiDatePicker::convertFormat"
– gipsy
2 days ago
@gipsy sorry my bad i usedDatePicker
instead ofDateTimePicker
i just updated it in the answer change it toDateTimePicker
and it will work
– Muhammad Omer Aslam
2 days ago
Thank you, now it works, it's just that the input is too complex, do you know other methods to create a model input field?
– gipsy
2 days ago
@gipsy what do you mean by input is too complex? and you can create with the above given methods when using a widget with input field , if its a normal field then you can also use theyiihelpersHtml
class too , for creating model inputs. do mark the answer as correct if it worked for you
– Muhammad Omer Aslam
2 days ago
add a comment |
If i understand correctly you are asking about using the widget with the model rather than calling it statically as you are currently copying the value to another ActiveForm field and then submitting it. If that is correct you can use the widget as a part of ActiveForm
field so that you don't have to insert the date into a separate model input to submit and save, see below.
<?php echo $form->field($model, 'opening_date')->widget(
DateTimePicker::class,
[
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]
);
?>
or you can pass the $model
and attribute
to your current code when calling the widget like below
echo DateTimePicker::widget(
[
'model' => $model,
'attribute' => 'opening_date',
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]
);
You can either pass the name
/model
& attribute
option see details
I tried with the first and this is the error: "Setting unknown property: yiijuiDatePicker::convertFormat"
– gipsy
2 days ago
@gipsy sorry my bad i usedDatePicker
instead ofDateTimePicker
i just updated it in the answer change it toDateTimePicker
and it will work
– Muhammad Omer Aslam
2 days ago
Thank you, now it works, it's just that the input is too complex, do you know other methods to create a model input field?
– gipsy
2 days ago
@gipsy what do you mean by input is too complex? and you can create with the above given methods when using a widget with input field , if its a normal field then you can also use theyiihelpersHtml
class too , for creating model inputs. do mark the answer as correct if it worked for you
– Muhammad Omer Aslam
2 days ago
add a comment |
If i understand correctly you are asking about using the widget with the model rather than calling it statically as you are currently copying the value to another ActiveForm field and then submitting it. If that is correct you can use the widget as a part of ActiveForm
field so that you don't have to insert the date into a separate model input to submit and save, see below.
<?php echo $form->field($model, 'opening_date')->widget(
DateTimePicker::class,
[
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]
);
?>
or you can pass the $model
and attribute
to your current code when calling the widget like below
echo DateTimePicker::widget(
[
'model' => $model,
'attribute' => 'opening_date',
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]
);
You can either pass the name
/model
& attribute
option see details
If i understand correctly you are asking about using the widget with the model rather than calling it statically as you are currently copying the value to another ActiveForm field and then submitting it. If that is correct you can use the widget as a part of ActiveForm
field so that you don't have to insert the date into a separate model input to submit and save, see below.
<?php echo $form->field($model, 'opening_date')->widget(
DateTimePicker::class,
[
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]
);
?>
or you can pass the $model
and attribute
to your current code when calling the widget like below
echo DateTimePicker::widget(
[
'model' => $model,
'attribute' => 'opening_date',
'options' => ['placeholder' => 'Select operating time ...'],
'convertFormat' => true,
'pluginOptions' => [
'format' => 'd-M-Y g:i A',
'startDate' => '01-Mar-2014 12:00 AM',
'todayHighlight' => true
]
]
);
You can either pass the name
/model
& attribute
option see details
edited 2 days ago
answered 2 days ago
Muhammad Omer AslamMuhammad Omer Aslam
12.5k62444
12.5k62444
I tried with the first and this is the error: "Setting unknown property: yiijuiDatePicker::convertFormat"
– gipsy
2 days ago
@gipsy sorry my bad i usedDatePicker
instead ofDateTimePicker
i just updated it in the answer change it toDateTimePicker
and it will work
– Muhammad Omer Aslam
2 days ago
Thank you, now it works, it's just that the input is too complex, do you know other methods to create a model input field?
– gipsy
2 days ago
@gipsy what do you mean by input is too complex? and you can create with the above given methods when using a widget with input field , if its a normal field then you can also use theyiihelpersHtml
class too , for creating model inputs. do mark the answer as correct if it worked for you
– Muhammad Omer Aslam
2 days ago
add a comment |
I tried with the first and this is the error: "Setting unknown property: yiijuiDatePicker::convertFormat"
– gipsy
2 days ago
@gipsy sorry my bad i usedDatePicker
instead ofDateTimePicker
i just updated it in the answer change it toDateTimePicker
and it will work
– Muhammad Omer Aslam
2 days ago
Thank you, now it works, it's just that the input is too complex, do you know other methods to create a model input field?
– gipsy
2 days ago
@gipsy what do you mean by input is too complex? and you can create with the above given methods when using a widget with input field , if its a normal field then you can also use theyiihelpersHtml
class too , for creating model inputs. do mark the answer as correct if it worked for you
– Muhammad Omer Aslam
2 days ago
I tried with the first and this is the error: "Setting unknown property: yiijuiDatePicker::convertFormat"
– gipsy
2 days ago
I tried with the first and this is the error: "Setting unknown property: yiijuiDatePicker::convertFormat"
– gipsy
2 days ago
@gipsy sorry my bad i used
DatePicker
instead of DateTimePicker
i just updated it in the answer change it to DateTimePicker
and it will work– Muhammad Omer Aslam
2 days ago
@gipsy sorry my bad i used
DatePicker
instead of DateTimePicker
i just updated it in the answer change it to DateTimePicker
and it will work– Muhammad Omer Aslam
2 days ago
Thank you, now it works, it's just that the input is too complex, do you know other methods to create a model input field?
– gipsy
2 days ago
Thank you, now it works, it's just that the input is too complex, do you know other methods to create a model input field?
– gipsy
2 days ago
@gipsy what do you mean by input is too complex? and you can create with the above given methods when using a widget with input field , if its a normal field then you can also use the
yiihelpersHtml
class too , for creating model inputs. do mark the answer as correct if it worked for you– Muhammad Omer Aslam
2 days ago
@gipsy what do you mean by input is too complex? and you can create with the above given methods when using a widget with input field , if its a normal field then you can also use the
yiihelpersHtml
class too , for creating model inputs. do mark the answer as correct if it worked for you– Muhammad Omer Aslam
2 days ago
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54252760%2fyii2-include-datetimepicker-in-activeform%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7JTXa1XxX3,QkUhBV,npl0HS,XnHOAxjK1o0sE2IR HLx w6R4h1odl3J2J,Kx6tY4pTRj,Y
ummmm... the question isnt clear you might need to provide more detail, why are you using a separate input field to submit the values when you are already using the widget, which also does the same?
– Muhammad Omer Aslam
2 days ago