firebase cloud function post request body
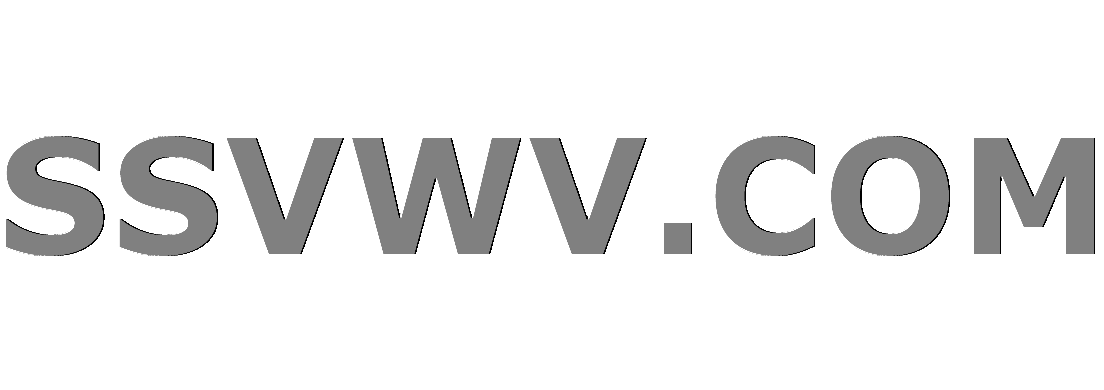
Multi tool use
I am making an http request from an angular form like that:
this.http.post(this.endPoint, formData, { responseType: 'text' }).subscribe(
res => {
console.log(res);
}
)
And I have a simple cloud function:
const functions = require('firebase-functions');
const cors = require('cors')({ origin: true });
exports.test = functions.https.onRequest((req, res) => {
cors(req, res, () => {
const data = req.body;
res.send(`Hello from Firebase! ${data}`);
});
})
However the req.body does not work and i get this response :
Hello from Firebase! [object Object]
Is there any idea why this happens?
node.js angular firebase google-cloud-functions
add a comment |
I am making an http request from an angular form like that:
this.http.post(this.endPoint, formData, { responseType: 'text' }).subscribe(
res => {
console.log(res);
}
)
And I have a simple cloud function:
const functions = require('firebase-functions');
const cors = require('cors')({ origin: true });
exports.test = functions.https.onRequest((req, res) => {
cors(req, res, () => {
const data = req.body;
res.send(`Hello from Firebase! ${data}`);
});
})
However the req.body does not work and i get this response :
Hello from Firebase! [object Object]
Is there any idea why this happens?
node.js angular firebase google-cloud-functions
add a comment |
I am making an http request from an angular form like that:
this.http.post(this.endPoint, formData, { responseType: 'text' }).subscribe(
res => {
console.log(res);
}
)
And I have a simple cloud function:
const functions = require('firebase-functions');
const cors = require('cors')({ origin: true });
exports.test = functions.https.onRequest((req, res) => {
cors(req, res, () => {
const data = req.body;
res.send(`Hello from Firebase! ${data}`);
});
})
However the req.body does not work and i get this response :
Hello from Firebase! [object Object]
Is there any idea why this happens?
node.js angular firebase google-cloud-functions
I am making an http request from an angular form like that:
this.http.post(this.endPoint, formData, { responseType: 'text' }).subscribe(
res => {
console.log(res);
}
)
And I have a simple cloud function:
const functions = require('firebase-functions');
const cors = require('cors')({ origin: true });
exports.test = functions.https.onRequest((req, res) => {
cors(req, res, () => {
const data = req.body;
res.send(`Hello from Firebase! ${data}`);
});
})
However the req.body does not work and i get this response :
Hello from Firebase! [object Object]
Is there any idea why this happens?
node.js angular firebase google-cloud-functions
node.js angular firebase google-cloud-functions
asked Jan 19 at 14:00
Giannis SavvidisGiannis Savvidis
1051112
1051112
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
If you're trying to print the req.body
value, you first have to convert it from a JavaScript object to a string:
res.send(`Hello from Firebase! ${JSON.stringify(data)}`);
add a comment |
Frank's answer is probably closer to what you are looking for.
Alternatively, if you're wanting to just print specific properties:
You are using the template literal to inject the req.body into your string. Since req.body (or data in this case) is an object, you'll have to pull the value(s) out of it that you want to display like req.body.prop
.
This example from Firebase quickstart shows pulling the property off the request body.
add a comment |
I found the problem, I added 'Content-Type': 'multipart/form-data'
in the headers when i post request from my angular form. And i tried to use busboy to parse the fields and this led me to another problem, it block the cors for some reason. Any ideas about that? Thanks by the way.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54267850%2ffirebase-cloud-function-post-request-body%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you're trying to print the req.body
value, you first have to convert it from a JavaScript object to a string:
res.send(`Hello from Firebase! ${JSON.stringify(data)}`);
add a comment |
If you're trying to print the req.body
value, you first have to convert it from a JavaScript object to a string:
res.send(`Hello from Firebase! ${JSON.stringify(data)}`);
add a comment |
If you're trying to print the req.body
value, you first have to convert it from a JavaScript object to a string:
res.send(`Hello from Firebase! ${JSON.stringify(data)}`);
If you're trying to print the req.body
value, you first have to convert it from a JavaScript object to a string:
res.send(`Hello from Firebase! ${JSON.stringify(data)}`);
answered Jan 19 at 14:34
Frank van PuffelenFrank van Puffelen
233k29380407
233k29380407
add a comment |
add a comment |
Frank's answer is probably closer to what you are looking for.
Alternatively, if you're wanting to just print specific properties:
You are using the template literal to inject the req.body into your string. Since req.body (or data in this case) is an object, you'll have to pull the value(s) out of it that you want to display like req.body.prop
.
This example from Firebase quickstart shows pulling the property off the request body.
add a comment |
Frank's answer is probably closer to what you are looking for.
Alternatively, if you're wanting to just print specific properties:
You are using the template literal to inject the req.body into your string. Since req.body (or data in this case) is an object, you'll have to pull the value(s) out of it that you want to display like req.body.prop
.
This example from Firebase quickstart shows pulling the property off the request body.
add a comment |
Frank's answer is probably closer to what you are looking for.
Alternatively, if you're wanting to just print specific properties:
You are using the template literal to inject the req.body into your string. Since req.body (or data in this case) is an object, you'll have to pull the value(s) out of it that you want to display like req.body.prop
.
This example from Firebase quickstart shows pulling the property off the request body.
Frank's answer is probably closer to what you are looking for.
Alternatively, if you're wanting to just print specific properties:
You are using the template literal to inject the req.body into your string. Since req.body (or data in this case) is an object, you'll have to pull the value(s) out of it that you want to display like req.body.prop
.
This example from Firebase quickstart shows pulling the property off the request body.
answered Jan 19 at 14:40


OneLunch ManOneLunch Man
34318
34318
add a comment |
add a comment |
I found the problem, I added 'Content-Type': 'multipart/form-data'
in the headers when i post request from my angular form. And i tried to use busboy to parse the fields and this led me to another problem, it block the cors for some reason. Any ideas about that? Thanks by the way.
add a comment |
I found the problem, I added 'Content-Type': 'multipart/form-data'
in the headers when i post request from my angular form. And i tried to use busboy to parse the fields and this led me to another problem, it block the cors for some reason. Any ideas about that? Thanks by the way.
add a comment |
I found the problem, I added 'Content-Type': 'multipart/form-data'
in the headers when i post request from my angular form. And i tried to use busboy to parse the fields and this led me to another problem, it block the cors for some reason. Any ideas about that? Thanks by the way.
I found the problem, I added 'Content-Type': 'multipart/form-data'
in the headers when i post request from my angular form. And i tried to use busboy to parse the fields and this led me to another problem, it block the cors for some reason. Any ideas about that? Thanks by the way.
answered Jan 19 at 15:12
Giannis SavvidisGiannis Savvidis
1051112
1051112
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54267850%2ffirebase-cloud-function-post-request-body%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
y663vz0kH63siu b J vpGWkIgH95d9x,M2pcozZLpTETbSx