What is the proper method to make close button of NbAlertComponent to work (close an alert)
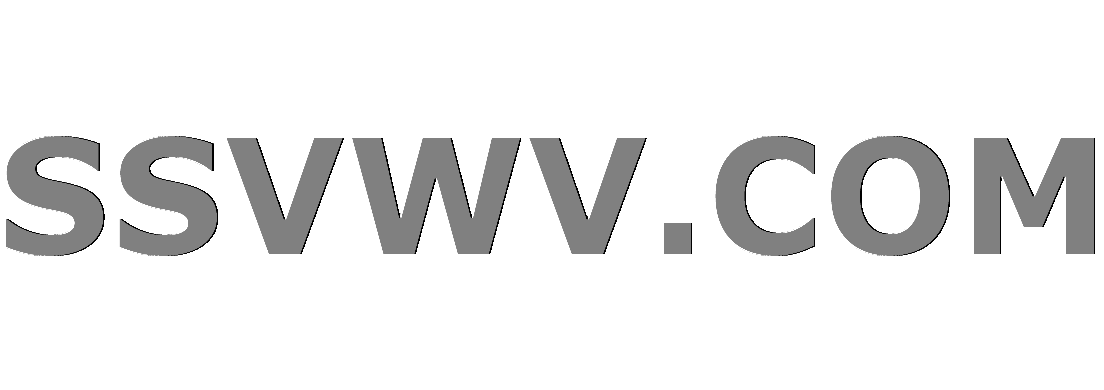
Multi tool use
I'm using nebular for my project, and cannot find out how to get close button
of NbAlertComponent to actually close alert. By closing I mean to stop showing after clicking on close button
. I read documentation about alert component docs, but did not found an answer. Alert component can have property closable
, which adds close button, and can have event handler when clicked on it (close)="onClose()"
. I'm using it this way (angular 6):
// page.component.html
<nb-alert status="success" closable (close)="onClose()">
You have been successfully authenticated!
</nb-alert>
In page.component.ts, if I have a method onClose
, it fires every time I click on alert close button
, but how to actually close it?
// page.component.ts
onClose() {
// fires after each click on close button:
console.log('close button was clicked');
}
Here is some code from alert component related close functionality:
// alert.component.ts
/**
* Emits when chip is removed
* @type EventEmitter<any>
*/
// this is an instance of NbAlertComponent
this.close = new EventEmitter();
/**
* Emits the removed chip event
*/
NbAlertComponent.prototype.onClose = function () {
this.close.emit();
};
javascript angular nebular
add a comment |
I'm using nebular for my project, and cannot find out how to get close button
of NbAlertComponent to actually close alert. By closing I mean to stop showing after clicking on close button
. I read documentation about alert component docs, but did not found an answer. Alert component can have property closable
, which adds close button, and can have event handler when clicked on it (close)="onClose()"
. I'm using it this way (angular 6):
// page.component.html
<nb-alert status="success" closable (close)="onClose()">
You have been successfully authenticated!
</nb-alert>
In page.component.ts, if I have a method onClose
, it fires every time I click on alert close button
, but how to actually close it?
// page.component.ts
onClose() {
// fires after each click on close button:
console.log('close button was clicked');
}
Here is some code from alert component related close functionality:
// alert.component.ts
/**
* Emits when chip is removed
* @type EventEmitter<any>
*/
// this is an instance of NbAlertComponent
this.close = new EventEmitter();
/**
* Emits the removed chip event
*/
NbAlertComponent.prototype.onClose = function () {
this.close.emit();
};
javascript angular nebular
1
use can simply create a css class that has display none property
– Thanveer Shah
Jan 19 at 14:07
@ThanveerShah, thanks, it's one of the way, which I end up to use
– Vadi
Jan 19 at 14:54
add a comment |
I'm using nebular for my project, and cannot find out how to get close button
of NbAlertComponent to actually close alert. By closing I mean to stop showing after clicking on close button
. I read documentation about alert component docs, but did not found an answer. Alert component can have property closable
, which adds close button, and can have event handler when clicked on it (close)="onClose()"
. I'm using it this way (angular 6):
// page.component.html
<nb-alert status="success" closable (close)="onClose()">
You have been successfully authenticated!
</nb-alert>
In page.component.ts, if I have a method onClose
, it fires every time I click on alert close button
, but how to actually close it?
// page.component.ts
onClose() {
// fires after each click on close button:
console.log('close button was clicked');
}
Here is some code from alert component related close functionality:
// alert.component.ts
/**
* Emits when chip is removed
* @type EventEmitter<any>
*/
// this is an instance of NbAlertComponent
this.close = new EventEmitter();
/**
* Emits the removed chip event
*/
NbAlertComponent.prototype.onClose = function () {
this.close.emit();
};
javascript angular nebular
I'm using nebular for my project, and cannot find out how to get close button
of NbAlertComponent to actually close alert. By closing I mean to stop showing after clicking on close button
. I read documentation about alert component docs, but did not found an answer. Alert component can have property closable
, which adds close button, and can have event handler when clicked on it (close)="onClose()"
. I'm using it this way (angular 6):
// page.component.html
<nb-alert status="success" closable (close)="onClose()">
You have been successfully authenticated!
</nb-alert>
In page.component.ts, if I have a method onClose
, it fires every time I click on alert close button
, but how to actually close it?
// page.component.ts
onClose() {
// fires after each click on close button:
console.log('close button was clicked');
}
Here is some code from alert component related close functionality:
// alert.component.ts
/**
* Emits when chip is removed
* @type EventEmitter<any>
*/
// this is an instance of NbAlertComponent
this.close = new EventEmitter();
/**
* Emits the removed chip event
*/
NbAlertComponent.prototype.onClose = function () {
this.close.emit();
};
javascript angular nebular
javascript angular nebular
edited Jan 19 at 14:10
Vadi
asked Jan 19 at 14:00
VadiVadi
39229
39229
1
use can simply create a css class that has display none property
– Thanveer Shah
Jan 19 at 14:07
@ThanveerShah, thanks, it's one of the way, which I end up to use
– Vadi
Jan 19 at 14:54
add a comment |
1
use can simply create a css class that has display none property
– Thanveer Shah
Jan 19 at 14:07
@ThanveerShah, thanks, it's one of the way, which I end up to use
– Vadi
Jan 19 at 14:54
1
1
use can simply create a css class that has display none property
– Thanveer Shah
Jan 19 at 14:07
use can simply create a css class that has display none property
– Thanveer Shah
Jan 19 at 14:07
@ThanveerShah, thanks, it's one of the way, which I end up to use
– Vadi
Jan 19 at 14:54
@ThanveerShah, thanks, it's one of the way, which I end up to use
– Vadi
Jan 19 at 14:54
add a comment |
2 Answers
2
active
oldest
votes
In this case you should be able to use the *ngIf
directive provided by Angular itself like so.
// page.component.html
<nb-alert status="success" closable (close)="onClose()" *ngIf="alertIsOpen">
You have been successfully authenticated!
</nb-alert>
alertIsOpen = true;
// page.component.ts
onClose() {
// fires after each click on close button:
console.log('close button was clicked');
this.alertIsOpen = false;
}
Another approach which would also work for multiple alerts would be to have your alerts exist in an array.
// page.component.html
<ng-container *ngFor="alert of alerts">
<nb-alert status="{{alert.status}}" closable (close)="onClose(alert)">
{{alert.text}}
</nb-alert>
</ng-container>
alerts = [
{
status: "success",
text: "You have been successfully authenticated!"
},
{
status: "danger",
text: "Failed to authenticate!"
}
]
// page.component.ts
onClose(alert) {
// fires after each click on close button:
console.log('close button was clicked');
this.alerts.splice(this.alerts.indexOf(alert), 1);
}
The benefit of these approaches are that you don't keep the alert existing inside your DOM
What if I have several alerts?
– Vadi
Jan 19 at 14:14
@Vadi I updated the answer with a solution that would allow for more alerts without having to separately defineisClosed
variables for them
– Jelle
Jan 19 at 19:29
add a comment |
End up with this solution:
// page.component.html
<nb-alert status="success" closable (click)="close($event)">
You have been successfully authenticated!
</nb-alert>
// page.component.ts
close($event) {
const closable = $event.target.closest("nb-alert.closable");
if (closable) {
closable.style.display = 'none';
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54267852%2fwhat-is-the-proper-method-to-make-close-button-of-nbalertcomponent-to-work-clos%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
In this case you should be able to use the *ngIf
directive provided by Angular itself like so.
// page.component.html
<nb-alert status="success" closable (close)="onClose()" *ngIf="alertIsOpen">
You have been successfully authenticated!
</nb-alert>
alertIsOpen = true;
// page.component.ts
onClose() {
// fires after each click on close button:
console.log('close button was clicked');
this.alertIsOpen = false;
}
Another approach which would also work for multiple alerts would be to have your alerts exist in an array.
// page.component.html
<ng-container *ngFor="alert of alerts">
<nb-alert status="{{alert.status}}" closable (close)="onClose(alert)">
{{alert.text}}
</nb-alert>
</ng-container>
alerts = [
{
status: "success",
text: "You have been successfully authenticated!"
},
{
status: "danger",
text: "Failed to authenticate!"
}
]
// page.component.ts
onClose(alert) {
// fires after each click on close button:
console.log('close button was clicked');
this.alerts.splice(this.alerts.indexOf(alert), 1);
}
The benefit of these approaches are that you don't keep the alert existing inside your DOM
What if I have several alerts?
– Vadi
Jan 19 at 14:14
@Vadi I updated the answer with a solution that would allow for more alerts without having to separately defineisClosed
variables for them
– Jelle
Jan 19 at 19:29
add a comment |
In this case you should be able to use the *ngIf
directive provided by Angular itself like so.
// page.component.html
<nb-alert status="success" closable (close)="onClose()" *ngIf="alertIsOpen">
You have been successfully authenticated!
</nb-alert>
alertIsOpen = true;
// page.component.ts
onClose() {
// fires after each click on close button:
console.log('close button was clicked');
this.alertIsOpen = false;
}
Another approach which would also work for multiple alerts would be to have your alerts exist in an array.
// page.component.html
<ng-container *ngFor="alert of alerts">
<nb-alert status="{{alert.status}}" closable (close)="onClose(alert)">
{{alert.text}}
</nb-alert>
</ng-container>
alerts = [
{
status: "success",
text: "You have been successfully authenticated!"
},
{
status: "danger",
text: "Failed to authenticate!"
}
]
// page.component.ts
onClose(alert) {
// fires after each click on close button:
console.log('close button was clicked');
this.alerts.splice(this.alerts.indexOf(alert), 1);
}
The benefit of these approaches are that you don't keep the alert existing inside your DOM
What if I have several alerts?
– Vadi
Jan 19 at 14:14
@Vadi I updated the answer with a solution that would allow for more alerts without having to separately defineisClosed
variables for them
– Jelle
Jan 19 at 19:29
add a comment |
In this case you should be able to use the *ngIf
directive provided by Angular itself like so.
// page.component.html
<nb-alert status="success" closable (close)="onClose()" *ngIf="alertIsOpen">
You have been successfully authenticated!
</nb-alert>
alertIsOpen = true;
// page.component.ts
onClose() {
// fires after each click on close button:
console.log('close button was clicked');
this.alertIsOpen = false;
}
Another approach which would also work for multiple alerts would be to have your alerts exist in an array.
// page.component.html
<ng-container *ngFor="alert of alerts">
<nb-alert status="{{alert.status}}" closable (close)="onClose(alert)">
{{alert.text}}
</nb-alert>
</ng-container>
alerts = [
{
status: "success",
text: "You have been successfully authenticated!"
},
{
status: "danger",
text: "Failed to authenticate!"
}
]
// page.component.ts
onClose(alert) {
// fires after each click on close button:
console.log('close button was clicked');
this.alerts.splice(this.alerts.indexOf(alert), 1);
}
The benefit of these approaches are that you don't keep the alert existing inside your DOM
In this case you should be able to use the *ngIf
directive provided by Angular itself like so.
// page.component.html
<nb-alert status="success" closable (close)="onClose()" *ngIf="alertIsOpen">
You have been successfully authenticated!
</nb-alert>
alertIsOpen = true;
// page.component.ts
onClose() {
// fires after each click on close button:
console.log('close button was clicked');
this.alertIsOpen = false;
}
Another approach which would also work for multiple alerts would be to have your alerts exist in an array.
// page.component.html
<ng-container *ngFor="alert of alerts">
<nb-alert status="{{alert.status}}" closable (close)="onClose(alert)">
{{alert.text}}
</nb-alert>
</ng-container>
alerts = [
{
status: "success",
text: "You have been successfully authenticated!"
},
{
status: "danger",
text: "Failed to authenticate!"
}
]
// page.component.ts
onClose(alert) {
// fires after each click on close button:
console.log('close button was clicked');
this.alerts.splice(this.alerts.indexOf(alert), 1);
}
The benefit of these approaches are that you don't keep the alert existing inside your DOM
edited Jan 19 at 19:28
answered Jan 19 at 14:10
JelleJelle
490110
490110
What if I have several alerts?
– Vadi
Jan 19 at 14:14
@Vadi I updated the answer with a solution that would allow for more alerts without having to separately defineisClosed
variables for them
– Jelle
Jan 19 at 19:29
add a comment |
What if I have several alerts?
– Vadi
Jan 19 at 14:14
@Vadi I updated the answer with a solution that would allow for more alerts without having to separately defineisClosed
variables for them
– Jelle
Jan 19 at 19:29
What if I have several alerts?
– Vadi
Jan 19 at 14:14
What if I have several alerts?
– Vadi
Jan 19 at 14:14
@Vadi I updated the answer with a solution that would allow for more alerts without having to separately define
isClosed
variables for them– Jelle
Jan 19 at 19:29
@Vadi I updated the answer with a solution that would allow for more alerts without having to separately define
isClosed
variables for them– Jelle
Jan 19 at 19:29
add a comment |
End up with this solution:
// page.component.html
<nb-alert status="success" closable (click)="close($event)">
You have been successfully authenticated!
</nb-alert>
// page.component.ts
close($event) {
const closable = $event.target.closest("nb-alert.closable");
if (closable) {
closable.style.display = 'none';
}
}
add a comment |
End up with this solution:
// page.component.html
<nb-alert status="success" closable (click)="close($event)">
You have been successfully authenticated!
</nb-alert>
// page.component.ts
close($event) {
const closable = $event.target.closest("nb-alert.closable");
if (closable) {
closable.style.display = 'none';
}
}
add a comment |
End up with this solution:
// page.component.html
<nb-alert status="success" closable (click)="close($event)">
You have been successfully authenticated!
</nb-alert>
// page.component.ts
close($event) {
const closable = $event.target.closest("nb-alert.closable");
if (closable) {
closable.style.display = 'none';
}
}
End up with this solution:
// page.component.html
<nb-alert status="success" closable (click)="close($event)">
You have been successfully authenticated!
</nb-alert>
// page.component.ts
close($event) {
const closable = $event.target.closest("nb-alert.closable");
if (closable) {
closable.style.display = 'none';
}
}
answered Jan 19 at 14:51
VadiVadi
39229
39229
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54267852%2fwhat-is-the-proper-method-to-make-close-button-of-nbalertcomponent-to-work-clos%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Scfc79uvAgPzJJKI X1N1
1
use can simply create a css class that has display none property
– Thanveer Shah
Jan 19 at 14:07
@ThanveerShah, thanks, it's one of the way, which I end up to use
– Vadi
Jan 19 at 14:54