R: How do I create a vector of user inputs into my function?
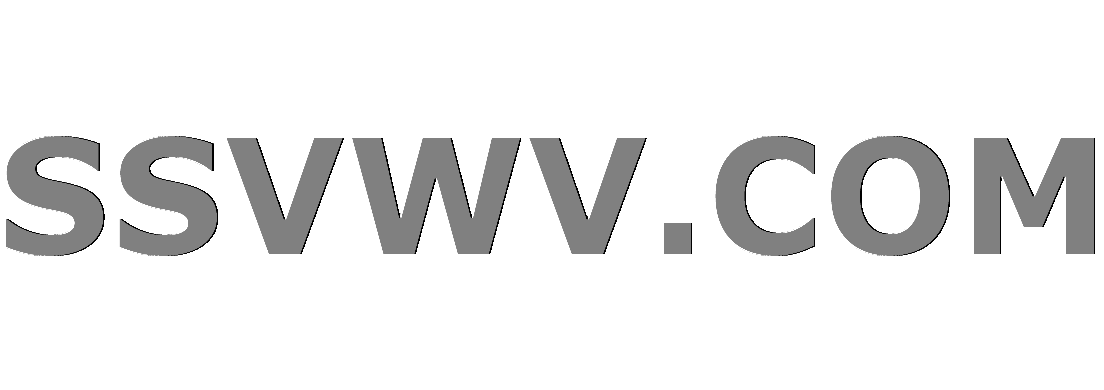
Multi tool use
I have this function for finding outliers. It works great, except when I make a mistake.
In that case, I need to go all the way back to the beginning.
The function plots the distribution for each variable in a data frame and asks for user input on the indexes related to the outliers.
This is the section of the function. It is embedded in a for loop.
#mark the extreme outliers, the rest are reasonable outliers
A <- colnames(df_out_id[i])
P <- readline(prompt="Would you like to see the full table of outliers? (enter to skip): ")
if(P == 0 | length(P) > 1){print("Reminder: the following questions identify outliers")}
if(P == "y" | P == "Y"){View(Outliers)}
W <- as.numeric(readline(prompt="Enter the index for first Extreme value on the lower limit (if none, enter 0): "))
Q <- as.numeric(readline(prompt="Enter the index for final Extreme value on the upper limit (if none, enter 0): "))
col <- df_out_id[i]
df_out_id[i] <- sapply(col[[1]], function(x){
if(Q>1 & x %in% Outliers$curr_column[1:Q]) return('Extreme')
if(W>1 & x %in% Outliers$curr_column[W:length(Outliers$curr_column)]) return('Extreme')
else if (x %in% Outliers$curr_column[Q+1:length(Outliers$curr_column)]) return('Reasonable')
else return('Non-Outlier')
})
}
#return a dataframe with outlier status, excluding the outlier ID columns
summary(df_out_id)
return(df_out_id[1:(length(names(df_out_id))-3)])
}
The full function is GetOutliers(), described here.
Is there a way to save any inputs to a vector and then print them at the end of the function?
Specifically, print them when the function successfully completes or in the case it is aborted along the way.
r
add a comment |
I have this function for finding outliers. It works great, except when I make a mistake.
In that case, I need to go all the way back to the beginning.
The function plots the distribution for each variable in a data frame and asks for user input on the indexes related to the outliers.
This is the section of the function. It is embedded in a for loop.
#mark the extreme outliers, the rest are reasonable outliers
A <- colnames(df_out_id[i])
P <- readline(prompt="Would you like to see the full table of outliers? (enter to skip): ")
if(P == 0 | length(P) > 1){print("Reminder: the following questions identify outliers")}
if(P == "y" | P == "Y"){View(Outliers)}
W <- as.numeric(readline(prompt="Enter the index for first Extreme value on the lower limit (if none, enter 0): "))
Q <- as.numeric(readline(prompt="Enter the index for final Extreme value on the upper limit (if none, enter 0): "))
col <- df_out_id[i]
df_out_id[i] <- sapply(col[[1]], function(x){
if(Q>1 & x %in% Outliers$curr_column[1:Q]) return('Extreme')
if(W>1 & x %in% Outliers$curr_column[W:length(Outliers$curr_column)]) return('Extreme')
else if (x %in% Outliers$curr_column[Q+1:length(Outliers$curr_column)]) return('Reasonable')
else return('Non-Outlier')
})
}
#return a dataframe with outlier status, excluding the outlier ID columns
summary(df_out_id)
return(df_out_id[1:(length(names(df_out_id))-3)])
}
The full function is GetOutliers(), described here.
Is there a way to save any inputs to a vector and then print them at the end of the function?
Specifically, print them when the function successfully completes or in the case it is aborted along the way.
r
add a comment |
I have this function for finding outliers. It works great, except when I make a mistake.
In that case, I need to go all the way back to the beginning.
The function plots the distribution for each variable in a data frame and asks for user input on the indexes related to the outliers.
This is the section of the function. It is embedded in a for loop.
#mark the extreme outliers, the rest are reasonable outliers
A <- colnames(df_out_id[i])
P <- readline(prompt="Would you like to see the full table of outliers? (enter to skip): ")
if(P == 0 | length(P) > 1){print("Reminder: the following questions identify outliers")}
if(P == "y" | P == "Y"){View(Outliers)}
W <- as.numeric(readline(prompt="Enter the index for first Extreme value on the lower limit (if none, enter 0): "))
Q <- as.numeric(readline(prompt="Enter the index for final Extreme value on the upper limit (if none, enter 0): "))
col <- df_out_id[i]
df_out_id[i] <- sapply(col[[1]], function(x){
if(Q>1 & x %in% Outliers$curr_column[1:Q]) return('Extreme')
if(W>1 & x %in% Outliers$curr_column[W:length(Outliers$curr_column)]) return('Extreme')
else if (x %in% Outliers$curr_column[Q+1:length(Outliers$curr_column)]) return('Reasonable')
else return('Non-Outlier')
})
}
#return a dataframe with outlier status, excluding the outlier ID columns
summary(df_out_id)
return(df_out_id[1:(length(names(df_out_id))-3)])
}
The full function is GetOutliers(), described here.
Is there a way to save any inputs to a vector and then print them at the end of the function?
Specifically, print them when the function successfully completes or in the case it is aborted along the way.
r
I have this function for finding outliers. It works great, except when I make a mistake.
In that case, I need to go all the way back to the beginning.
The function plots the distribution for each variable in a data frame and asks for user input on the indexes related to the outliers.
This is the section of the function. It is embedded in a for loop.
#mark the extreme outliers, the rest are reasonable outliers
A <- colnames(df_out_id[i])
P <- readline(prompt="Would you like to see the full table of outliers? (enter to skip): ")
if(P == 0 | length(P) > 1){print("Reminder: the following questions identify outliers")}
if(P == "y" | P == "Y"){View(Outliers)}
W <- as.numeric(readline(prompt="Enter the index for first Extreme value on the lower limit (if none, enter 0): "))
Q <- as.numeric(readline(prompt="Enter the index for final Extreme value on the upper limit (if none, enter 0): "))
col <- df_out_id[i]
df_out_id[i] <- sapply(col[[1]], function(x){
if(Q>1 & x %in% Outliers$curr_column[1:Q]) return('Extreme')
if(W>1 & x %in% Outliers$curr_column[W:length(Outliers$curr_column)]) return('Extreme')
else if (x %in% Outliers$curr_column[Q+1:length(Outliers$curr_column)]) return('Reasonable')
else return('Non-Outlier')
})
}
#return a dataframe with outlier status, excluding the outlier ID columns
summary(df_out_id)
return(df_out_id[1:(length(names(df_out_id))-3)])
}
The full function is GetOutliers(), described here.
Is there a way to save any inputs to a vector and then print them at the end of the function?
Specifically, print them when the function successfully completes or in the case it is aborted along the way.
r
r
asked Jan 20 at 3:22
SebastianSebastian
185112
185112
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54273330%2fr-how-do-i-create-a-vector-of-user-inputs-into-my-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54273330%2fr-how-do-i-create-a-vector-of-user-inputs-into-my-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
aAl00Ud2bXacLj6jFLIwPIrsvcl4R,QIL x5iJrtv1mDAWYsDy0XJbIJjJUz5dgz hYCT4WjVgPjrZn