Keep updating list while summing
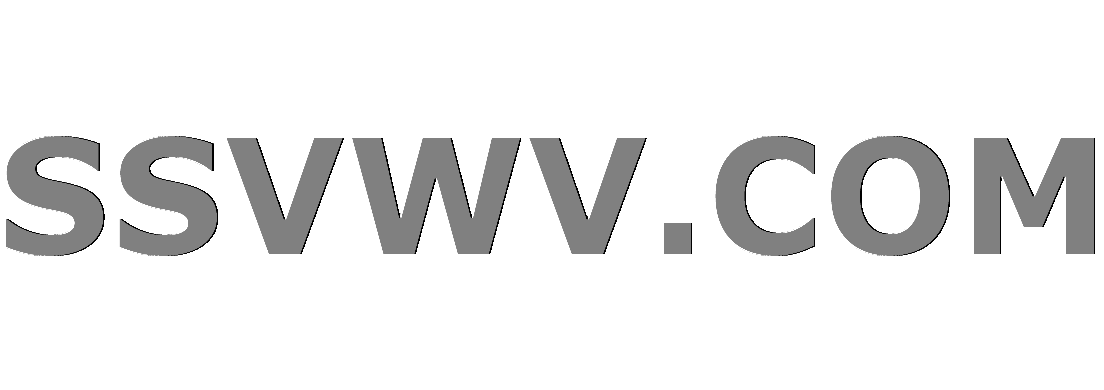
Multi tool use
I'm a begginer in Python. I need help.
I recieve a starting point (in this case, (2, 0)) and must sum 2 numbers to that. Eg (2, 0) + (1, 1) = (3, 1).
I have to keep doing this till I achieve a maximum value (in this case 5) of elements is the list.
But I have a problem. I can't make a loop and keep updating the list.
The function must work with any value. Can someone help me?
Here is what I have (know it's wrong, but i'm stuck):
def summ(a, b):
return (a[0] + b[0], a[1] + b[1])
x= (((5), (2,0), (1,1)))
maxx= x[0] #max of elements in list
start= x[1] #starting point
direction= x[2] #what it must sum
def position(x):
new_list= ()
for i in x:
new_list = start, summ(start, direction)
new_list += (summ(new_list[-1], direction), )
if len(new_list) == maxx:
break
return new_list
#the output I had
((2, 0), (3, 1), (4, 2))
#the output I need
((2, 0), (3, 1), (4, 2), (5, 3), (6, 4)) #5 elements, maxx
python
add a comment |
I'm a begginer in Python. I need help.
I recieve a starting point (in this case, (2, 0)) and must sum 2 numbers to that. Eg (2, 0) + (1, 1) = (3, 1).
I have to keep doing this till I achieve a maximum value (in this case 5) of elements is the list.
But I have a problem. I can't make a loop and keep updating the list.
The function must work with any value. Can someone help me?
Here is what I have (know it's wrong, but i'm stuck):
def summ(a, b):
return (a[0] + b[0], a[1] + b[1])
x= (((5), (2,0), (1,1)))
maxx= x[0] #max of elements in list
start= x[1] #starting point
direction= x[2] #what it must sum
def position(x):
new_list= ()
for i in x:
new_list = start, summ(start, direction)
new_list += (summ(new_list[-1], direction), )
if len(new_list) == maxx:
break
return new_list
#the output I had
((2, 0), (3, 1), (4, 2))
#the output I need
((2, 0), (3, 1), (4, 2), (5, 3), (6, 4)) #5 elements, maxx
python
1
move thenew_list = start, summ(start, direction)
outside of the for loop.
– Stephen Rauch
Jan 19 at 3:03
add a comment |
I'm a begginer in Python. I need help.
I recieve a starting point (in this case, (2, 0)) and must sum 2 numbers to that. Eg (2, 0) + (1, 1) = (3, 1).
I have to keep doing this till I achieve a maximum value (in this case 5) of elements is the list.
But I have a problem. I can't make a loop and keep updating the list.
The function must work with any value. Can someone help me?
Here is what I have (know it's wrong, but i'm stuck):
def summ(a, b):
return (a[0] + b[0], a[1] + b[1])
x= (((5), (2,0), (1,1)))
maxx= x[0] #max of elements in list
start= x[1] #starting point
direction= x[2] #what it must sum
def position(x):
new_list= ()
for i in x:
new_list = start, summ(start, direction)
new_list += (summ(new_list[-1], direction), )
if len(new_list) == maxx:
break
return new_list
#the output I had
((2, 0), (3, 1), (4, 2))
#the output I need
((2, 0), (3, 1), (4, 2), (5, 3), (6, 4)) #5 elements, maxx
python
I'm a begginer in Python. I need help.
I recieve a starting point (in this case, (2, 0)) and must sum 2 numbers to that. Eg (2, 0) + (1, 1) = (3, 1).
I have to keep doing this till I achieve a maximum value (in this case 5) of elements is the list.
But I have a problem. I can't make a loop and keep updating the list.
The function must work with any value. Can someone help me?
Here is what I have (know it's wrong, but i'm stuck):
def summ(a, b):
return (a[0] + b[0], a[1] + b[1])
x= (((5), (2,0), (1,1)))
maxx= x[0] #max of elements in list
start= x[1] #starting point
direction= x[2] #what it must sum
def position(x):
new_list= ()
for i in x:
new_list = start, summ(start, direction)
new_list += (summ(new_list[-1], direction), )
if len(new_list) == maxx:
break
return new_list
#the output I had
((2, 0), (3, 1), (4, 2))
#the output I need
((2, 0), (3, 1), (4, 2), (5, 3), (6, 4)) #5 elements, maxx
python
python
asked Jan 19 at 2:57
Kelly BaptistaKelly Baptista
54
54
1
move thenew_list = start, summ(start, direction)
outside of the for loop.
– Stephen Rauch
Jan 19 at 3:03
add a comment |
1
move thenew_list = start, summ(start, direction)
outside of the for loop.
– Stephen Rauch
Jan 19 at 3:03
1
1
move the
new_list = start, summ(start, direction)
outside of the for loop.– Stephen Rauch
Jan 19 at 3:03
move the
new_list = start, summ(start, direction)
outside of the for loop.– Stephen Rauch
Jan 19 at 3:03
add a comment |
3 Answers
3
active
oldest
votes
def summ(a, b):
return a[0] + b[0], a[1] + b[1]
x = 5, (2, 0), (1, 1)
def position(x):
i = 1
new_list = [x[i]]
while i < x[0]:
new_list += summ(new_list[i - 1], x[2]),
i = i + 1
return new_list
print(position(x))
Tested and the OUTPUT is
[(2, 0), (3, 1), (4, 2), (5, 3), (6, 4)]
1
I just added to the return: tuple(new_list). So the output will be ((2,0), (3,1), (4,2), (5,3), (6,4)). Thank you! :)
– Kelly Baptista
Jan 19 at 19:50
add a comment |
Use a list comprehension:
a = ((5,), (2,0), (1,1))
b = [(a[1][0] + i*a[2][0], a[1][1] + i*a[2][1]) for i in range(a[0][0])]
add a comment |
Let's take @StephenRauch's sage advice and move the:
new_list = start, summ(start, direction)
outside of the for
loop. And clean up the code as we do:
def summ(a, b):
return a[0] + b[0], a[1] + b[1]
def position(argument):
maxx, start, direction = argument
new_list = [start]
for _ in range(maxx - len(new_list)):
new_list.append(summ(new_list[-1], direction))
return tuple(new_list)
print(position(((5), (2, 0), (1, 1))))
OUTPUT
> python3 test.py
((2, 0), (3, 1), (4, 2), (5, 3), (6, 4))
>
Now let's have some more fun with the code and rather than reinvent vector arithmetic, we'll import Vec2D
from Python turtle. And return a list
which makes more sense to me than a tuple
:
from turtle import Vec2D
def position(argument):
maxx, start, direction = argument
return [start + n * direction for n in range(maxx)]
print(position(((5), Vec2D(2, 0), Vec2D(1, 1))))
OUTPUT
> python3 test2.py
[(2.00,0.00), (3.00,1.00), (4.00,2.00), (5.00,3.00), (6.00,4.00)]
>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54263707%2fkeep-updating-list-while-summing%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
def summ(a, b):
return a[0] + b[0], a[1] + b[1]
x = 5, (2, 0), (1, 1)
def position(x):
i = 1
new_list = [x[i]]
while i < x[0]:
new_list += summ(new_list[i - 1], x[2]),
i = i + 1
return new_list
print(position(x))
Tested and the OUTPUT is
[(2, 0), (3, 1), (4, 2), (5, 3), (6, 4)]
1
I just added to the return: tuple(new_list). So the output will be ((2,0), (3,1), (4,2), (5,3), (6,4)). Thank you! :)
– Kelly Baptista
Jan 19 at 19:50
add a comment |
def summ(a, b):
return a[0] + b[0], a[1] + b[1]
x = 5, (2, 0), (1, 1)
def position(x):
i = 1
new_list = [x[i]]
while i < x[0]:
new_list += summ(new_list[i - 1], x[2]),
i = i + 1
return new_list
print(position(x))
Tested and the OUTPUT is
[(2, 0), (3, 1), (4, 2), (5, 3), (6, 4)]
1
I just added to the return: tuple(new_list). So the output will be ((2,0), (3,1), (4,2), (5,3), (6,4)). Thank you! :)
– Kelly Baptista
Jan 19 at 19:50
add a comment |
def summ(a, b):
return a[0] + b[0], a[1] + b[1]
x = 5, (2, 0), (1, 1)
def position(x):
i = 1
new_list = [x[i]]
while i < x[0]:
new_list += summ(new_list[i - 1], x[2]),
i = i + 1
return new_list
print(position(x))
Tested and the OUTPUT is
[(2, 0), (3, 1), (4, 2), (5, 3), (6, 4)]
def summ(a, b):
return a[0] + b[0], a[1] + b[1]
x = 5, (2, 0), (1, 1)
def position(x):
i = 1
new_list = [x[i]]
while i < x[0]:
new_list += summ(new_list[i - 1], x[2]),
i = i + 1
return new_list
print(position(x))
Tested and the OUTPUT is
[(2, 0), (3, 1), (4, 2), (5, 3), (6, 4)]
edited Jan 19 at 4:15
answered Jan 19 at 3:36


Hassan VoyeauHassan Voyeau
1,69021720
1,69021720
1
I just added to the return: tuple(new_list). So the output will be ((2,0), (3,1), (4,2), (5,3), (6,4)). Thank you! :)
– Kelly Baptista
Jan 19 at 19:50
add a comment |
1
I just added to the return: tuple(new_list). So the output will be ((2,0), (3,1), (4,2), (5,3), (6,4)). Thank you! :)
– Kelly Baptista
Jan 19 at 19:50
1
1
I just added to the return: tuple(new_list). So the output will be ((2,0), (3,1), (4,2), (5,3), (6,4)). Thank you! :)
– Kelly Baptista
Jan 19 at 19:50
I just added to the return: tuple(new_list). So the output will be ((2,0), (3,1), (4,2), (5,3), (6,4)). Thank you! :)
– Kelly Baptista
Jan 19 at 19:50
add a comment |
Use a list comprehension:
a = ((5,), (2,0), (1,1))
b = [(a[1][0] + i*a[2][0], a[1][1] + i*a[2][1]) for i in range(a[0][0])]
add a comment |
Use a list comprehension:
a = ((5,), (2,0), (1,1))
b = [(a[1][0] + i*a[2][0], a[1][1] + i*a[2][1]) for i in range(a[0][0])]
add a comment |
Use a list comprehension:
a = ((5,), (2,0), (1,1))
b = [(a[1][0] + i*a[2][0], a[1][1] + i*a[2][1]) for i in range(a[0][0])]
Use a list comprehension:
a = ((5,), (2,0), (1,1))
b = [(a[1][0] + i*a[2][0], a[1][1] + i*a[2][1]) for i in range(a[0][0])]
edited Jan 19 at 10:21
answered Jan 19 at 9:43
Jan Christoph TerasaJan Christoph Terasa
1,9501022
1,9501022
add a comment |
add a comment |
Let's take @StephenRauch's sage advice and move the:
new_list = start, summ(start, direction)
outside of the for
loop. And clean up the code as we do:
def summ(a, b):
return a[0] + b[0], a[1] + b[1]
def position(argument):
maxx, start, direction = argument
new_list = [start]
for _ in range(maxx - len(new_list)):
new_list.append(summ(new_list[-1], direction))
return tuple(new_list)
print(position(((5), (2, 0), (1, 1))))
OUTPUT
> python3 test.py
((2, 0), (3, 1), (4, 2), (5, 3), (6, 4))
>
Now let's have some more fun with the code and rather than reinvent vector arithmetic, we'll import Vec2D
from Python turtle. And return a list
which makes more sense to me than a tuple
:
from turtle import Vec2D
def position(argument):
maxx, start, direction = argument
return [start + n * direction for n in range(maxx)]
print(position(((5), Vec2D(2, 0), Vec2D(1, 1))))
OUTPUT
> python3 test2.py
[(2.00,0.00), (3.00,1.00), (4.00,2.00), (5.00,3.00), (6.00,4.00)]
>
add a comment |
Let's take @StephenRauch's sage advice and move the:
new_list = start, summ(start, direction)
outside of the for
loop. And clean up the code as we do:
def summ(a, b):
return a[0] + b[0], a[1] + b[1]
def position(argument):
maxx, start, direction = argument
new_list = [start]
for _ in range(maxx - len(new_list)):
new_list.append(summ(new_list[-1], direction))
return tuple(new_list)
print(position(((5), (2, 0), (1, 1))))
OUTPUT
> python3 test.py
((2, 0), (3, 1), (4, 2), (5, 3), (6, 4))
>
Now let's have some more fun with the code and rather than reinvent vector arithmetic, we'll import Vec2D
from Python turtle. And return a list
which makes more sense to me than a tuple
:
from turtle import Vec2D
def position(argument):
maxx, start, direction = argument
return [start + n * direction for n in range(maxx)]
print(position(((5), Vec2D(2, 0), Vec2D(1, 1))))
OUTPUT
> python3 test2.py
[(2.00,0.00), (3.00,1.00), (4.00,2.00), (5.00,3.00), (6.00,4.00)]
>
add a comment |
Let's take @StephenRauch's sage advice and move the:
new_list = start, summ(start, direction)
outside of the for
loop. And clean up the code as we do:
def summ(a, b):
return a[0] + b[0], a[1] + b[1]
def position(argument):
maxx, start, direction = argument
new_list = [start]
for _ in range(maxx - len(new_list)):
new_list.append(summ(new_list[-1], direction))
return tuple(new_list)
print(position(((5), (2, 0), (1, 1))))
OUTPUT
> python3 test.py
((2, 0), (3, 1), (4, 2), (5, 3), (6, 4))
>
Now let's have some more fun with the code and rather than reinvent vector arithmetic, we'll import Vec2D
from Python turtle. And return a list
which makes more sense to me than a tuple
:
from turtle import Vec2D
def position(argument):
maxx, start, direction = argument
return [start + n * direction for n in range(maxx)]
print(position(((5), Vec2D(2, 0), Vec2D(1, 1))))
OUTPUT
> python3 test2.py
[(2.00,0.00), (3.00,1.00), (4.00,2.00), (5.00,3.00), (6.00,4.00)]
>
Let's take @StephenRauch's sage advice and move the:
new_list = start, summ(start, direction)
outside of the for
loop. And clean up the code as we do:
def summ(a, b):
return a[0] + b[0], a[1] + b[1]
def position(argument):
maxx, start, direction = argument
new_list = [start]
for _ in range(maxx - len(new_list)):
new_list.append(summ(new_list[-1], direction))
return tuple(new_list)
print(position(((5), (2, 0), (1, 1))))
OUTPUT
> python3 test.py
((2, 0), (3, 1), (4, 2), (5, 3), (6, 4))
>
Now let's have some more fun with the code and rather than reinvent vector arithmetic, we'll import Vec2D
from Python turtle. And return a list
which makes more sense to me than a tuple
:
from turtle import Vec2D
def position(argument):
maxx, start, direction = argument
return [start + n * direction for n in range(maxx)]
print(position(((5), Vec2D(2, 0), Vec2D(1, 1))))
OUTPUT
> python3 test2.py
[(2.00,0.00), (3.00,1.00), (4.00,2.00), (5.00,3.00), (6.00,4.00)]
>
edited Jan 20 at 22:20
answered Jan 19 at 6:04
cdlanecdlane
17.9k21144
17.9k21144
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54263707%2fkeep-updating-list-while-summing%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QYRV0zxzbHKUI
1
move the
new_list = start, summ(start, direction)
outside of the for loop.– Stephen Rauch
Jan 19 at 3:03