Data type error for tf.data.Dataset.from_tensor_slices… Cannot convert a TensorShape to dtype:
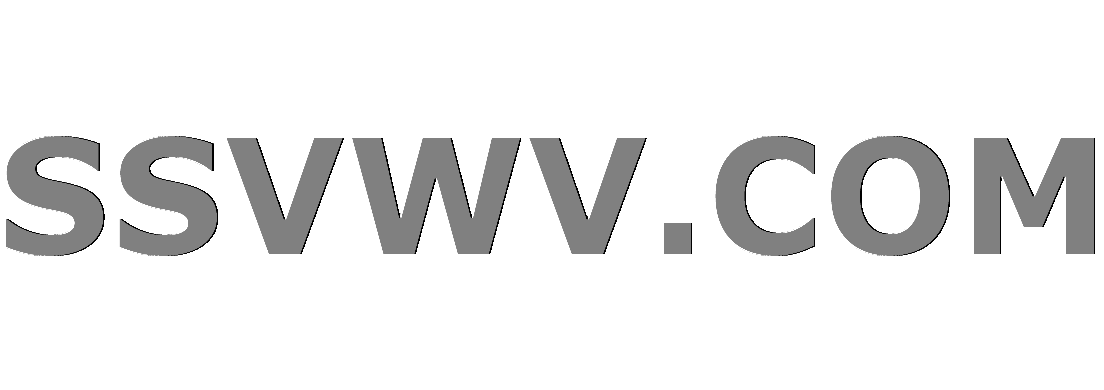
Multi tool use
I'm trying to take data from a csv with a list of files and a list of labels, and convert it to being one-hot labeled for a categorical classification using tf.keras. I am using eager mode for the code.
I'm trying to follow the tf.data example from CS230 building a data pipeline.
https://cs230-stanford.github.io/tensorflow-input-data.html
my code is below under the code section.
the csv file that lists the location of all the pictures is located on dropbox here:
https://www.dropbox.com/s/5uo8o1p30g2aeta/Clock.csv?dl=0
When I run the code as shown below I get a
TypeError: Cannot convert a TensorShape to dtype: <dtype: 'float32'>
error.
When I add to line 55 and make line 56 :
one_hot_Hr = tf.one_hot(file.Hr,classes)
one_hot_Hr = tf.to_int32(one_hot_Hr)
I get this error:
InvalidArgumentError: cannot compute Mul as input #0 was expected to be
a float tensor but is a int32 tensor [Op:Mul]
name: loss/activation_2_loss/mul/
when I run
iterator.get_next()
the pictures are formated as
<tf.Tensor: id=12462, shape=(32, 300, 300, 3), dtype=float32, numpy=
the labels are formated as:
<tf.Tensor: id=12463, shape=(32, 13), dtype=float32, numpy=
based on the errors, it seems like it should be a simple formatting issue with the labels, but I'm stumped and neither error brings up much useful information on stack overflow.
Code:
import pandas as pd
import tensorflow as tf
import tensorflow.keras as k
#import cv2
#tf.enable_eager_execution()
#import argparse
#from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras.layers import Conv2D, MaxPooling2D
from tensorflow.keras.layers import Activation, Dropout, Flatten, Dense
def parse_function(filename, label):
image_string = tf.read_file(filename)
# Don't use tf.image.decode_image, or the output shape will be undefined
image = tf.image.decode_jpeg(image_string, channels=3)
# This will convert to float values in [0, 1]
image = tf.image.convert_image_dtype(image, tf.float32)
image = tf.image.resize_images(image, [300, 300])
return image, label
def train_preprocess(image, label):
image = tf.image.random_flip_left_right(image)
image = tf.image.random_brightness(image, max_delta=32.0 / 255.0)
image = tf.image.random_saturation(image, lower=0.5, upper=1.5)
# Make sure the image is still in [0, 1]
image = tf.clip_by_value(image, 0.0, 1.0)
return image, label
batch_size = 32
classes = 13
fileLoc = "C:/Users/USAgData/TF/Clock.csv"
file = pd.read_csv(fileLoc)
file['Loc']=''
file.Loc = str(str(file.Location)[9:23] + str(file.Location)[28:46])
one_hot_Hr = tf.one_hot(file.Hr,classes)
#one_hot_Hr = tf.to_int32(one_hot_Hr)
dataset = tf.data.Dataset.from_tensor_slices((file.Loc, one_hot_Hr))
dataset = dataset.shuffle(len(file.Location))
dataset = dataset.map(parse_function, num_parallel_calls=4)
dataset = dataset.map(train_preprocess, num_parallel_calls=4)
dataset = dataset.batch(batch_size)
dataset = dataset.prefetch(1)
#print(dataset.shape) # ==> "(tf.float32, tf.float32)"
iterator = dataset.make_one_shot_iterator()
next_element = iterator.get_next()
#print(next_element)
tf.keras.backend.clear_session()
model_name="Documentation"
model = k.Sequential()
model.add(Conv2D(64, (3, 3), input_shape=(300,300,3))) #Changed shape to include batch
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
#model.add(Conv2D(32, (3, 3)))
#model.add(Activation('relu'))
#model.add(MaxPooling2D(pool_size=(2, 2)))
#model.add(Conv2D(64, (3, 3)))
#model.add(Activation('relu'))
#model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten())
model.add(Dense(32))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(classes))
model.add(Activation('softmax')) #Changed from sigmoid
#changed from categorical cross entropy
model.compile(loss='categorical_crossentropy',
optimizer=tf.train.RMSPropOptimizer(.0001),
metrics=['accuracy'])
model.summary()
fitting = model.fit_generator(iterator,epochs =1 ,shuffle=False, steps_per_epoch=14400//batch_size)
#model.evaluate(dataset,steps=30)
import sys
print(sys.version)
tf.__version__
I'm running:
tf: 1.10.0
Python: 3.6.7 |Anaconda custom (64-bit)| (default, Dec 10 2018, 20:35:02) [MSC v.1915 64 bit (AMD64)]
I don't know if this should truly be the solution, but when I switch:
fitting = model.fit_generator(iterator,epochs =1 ,shuffle=False, steps_per_epoch=14400//batch_size)
to
fitting = model.fit(iterator,epochs = 1 , shuffle = False, steps_per_epoch = 14400//batch_size)
The model does start to train. But, then them model runs out of data points because the iterator will not start over again.
python tensorflow keras tensorflow-datasets
add a comment |
I'm trying to take data from a csv with a list of files and a list of labels, and convert it to being one-hot labeled for a categorical classification using tf.keras. I am using eager mode for the code.
I'm trying to follow the tf.data example from CS230 building a data pipeline.
https://cs230-stanford.github.io/tensorflow-input-data.html
my code is below under the code section.
the csv file that lists the location of all the pictures is located on dropbox here:
https://www.dropbox.com/s/5uo8o1p30g2aeta/Clock.csv?dl=0
When I run the code as shown below I get a
TypeError: Cannot convert a TensorShape to dtype: <dtype: 'float32'>
error.
When I add to line 55 and make line 56 :
one_hot_Hr = tf.one_hot(file.Hr,classes)
one_hot_Hr = tf.to_int32(one_hot_Hr)
I get this error:
InvalidArgumentError: cannot compute Mul as input #0 was expected to be
a float tensor but is a int32 tensor [Op:Mul]
name: loss/activation_2_loss/mul/
when I run
iterator.get_next()
the pictures are formated as
<tf.Tensor: id=12462, shape=(32, 300, 300, 3), dtype=float32, numpy=
the labels are formated as:
<tf.Tensor: id=12463, shape=(32, 13), dtype=float32, numpy=
based on the errors, it seems like it should be a simple formatting issue with the labels, but I'm stumped and neither error brings up much useful information on stack overflow.
Code:
import pandas as pd
import tensorflow as tf
import tensorflow.keras as k
#import cv2
#tf.enable_eager_execution()
#import argparse
#from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras.layers import Conv2D, MaxPooling2D
from tensorflow.keras.layers import Activation, Dropout, Flatten, Dense
def parse_function(filename, label):
image_string = tf.read_file(filename)
# Don't use tf.image.decode_image, or the output shape will be undefined
image = tf.image.decode_jpeg(image_string, channels=3)
# This will convert to float values in [0, 1]
image = tf.image.convert_image_dtype(image, tf.float32)
image = tf.image.resize_images(image, [300, 300])
return image, label
def train_preprocess(image, label):
image = tf.image.random_flip_left_right(image)
image = tf.image.random_brightness(image, max_delta=32.0 / 255.0)
image = tf.image.random_saturation(image, lower=0.5, upper=1.5)
# Make sure the image is still in [0, 1]
image = tf.clip_by_value(image, 0.0, 1.0)
return image, label
batch_size = 32
classes = 13
fileLoc = "C:/Users/USAgData/TF/Clock.csv"
file = pd.read_csv(fileLoc)
file['Loc']=''
file.Loc = str(str(file.Location)[9:23] + str(file.Location)[28:46])
one_hot_Hr = tf.one_hot(file.Hr,classes)
#one_hot_Hr = tf.to_int32(one_hot_Hr)
dataset = tf.data.Dataset.from_tensor_slices((file.Loc, one_hot_Hr))
dataset = dataset.shuffle(len(file.Location))
dataset = dataset.map(parse_function, num_parallel_calls=4)
dataset = dataset.map(train_preprocess, num_parallel_calls=4)
dataset = dataset.batch(batch_size)
dataset = dataset.prefetch(1)
#print(dataset.shape) # ==> "(tf.float32, tf.float32)"
iterator = dataset.make_one_shot_iterator()
next_element = iterator.get_next()
#print(next_element)
tf.keras.backend.clear_session()
model_name="Documentation"
model = k.Sequential()
model.add(Conv2D(64, (3, 3), input_shape=(300,300,3))) #Changed shape to include batch
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
#model.add(Conv2D(32, (3, 3)))
#model.add(Activation('relu'))
#model.add(MaxPooling2D(pool_size=(2, 2)))
#model.add(Conv2D(64, (3, 3)))
#model.add(Activation('relu'))
#model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten())
model.add(Dense(32))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(classes))
model.add(Activation('softmax')) #Changed from sigmoid
#changed from categorical cross entropy
model.compile(loss='categorical_crossentropy',
optimizer=tf.train.RMSPropOptimizer(.0001),
metrics=['accuracy'])
model.summary()
fitting = model.fit_generator(iterator,epochs =1 ,shuffle=False, steps_per_epoch=14400//batch_size)
#model.evaluate(dataset,steps=30)
import sys
print(sys.version)
tf.__version__
I'm running:
tf: 1.10.0
Python: 3.6.7 |Anaconda custom (64-bit)| (default, Dec 10 2018, 20:35:02) [MSC v.1915 64 bit (AMD64)]
I don't know if this should truly be the solution, but when I switch:
fitting = model.fit_generator(iterator,epochs =1 ,shuffle=False, steps_per_epoch=14400//batch_size)
to
fitting = model.fit(iterator,epochs = 1 , shuffle = False, steps_per_epoch = 14400//batch_size)
The model does start to train. But, then them model runs out of data points because the iterator will not start over again.
python tensorflow keras tensorflow-datasets
add a comment |
I'm trying to take data from a csv with a list of files and a list of labels, and convert it to being one-hot labeled for a categorical classification using tf.keras. I am using eager mode for the code.
I'm trying to follow the tf.data example from CS230 building a data pipeline.
https://cs230-stanford.github.io/tensorflow-input-data.html
my code is below under the code section.
the csv file that lists the location of all the pictures is located on dropbox here:
https://www.dropbox.com/s/5uo8o1p30g2aeta/Clock.csv?dl=0
When I run the code as shown below I get a
TypeError: Cannot convert a TensorShape to dtype: <dtype: 'float32'>
error.
When I add to line 55 and make line 56 :
one_hot_Hr = tf.one_hot(file.Hr,classes)
one_hot_Hr = tf.to_int32(one_hot_Hr)
I get this error:
InvalidArgumentError: cannot compute Mul as input #0 was expected to be
a float tensor but is a int32 tensor [Op:Mul]
name: loss/activation_2_loss/mul/
when I run
iterator.get_next()
the pictures are formated as
<tf.Tensor: id=12462, shape=(32, 300, 300, 3), dtype=float32, numpy=
the labels are formated as:
<tf.Tensor: id=12463, shape=(32, 13), dtype=float32, numpy=
based on the errors, it seems like it should be a simple formatting issue with the labels, but I'm stumped and neither error brings up much useful information on stack overflow.
Code:
import pandas as pd
import tensorflow as tf
import tensorflow.keras as k
#import cv2
#tf.enable_eager_execution()
#import argparse
#from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras.layers import Conv2D, MaxPooling2D
from tensorflow.keras.layers import Activation, Dropout, Flatten, Dense
def parse_function(filename, label):
image_string = tf.read_file(filename)
# Don't use tf.image.decode_image, or the output shape will be undefined
image = tf.image.decode_jpeg(image_string, channels=3)
# This will convert to float values in [0, 1]
image = tf.image.convert_image_dtype(image, tf.float32)
image = tf.image.resize_images(image, [300, 300])
return image, label
def train_preprocess(image, label):
image = tf.image.random_flip_left_right(image)
image = tf.image.random_brightness(image, max_delta=32.0 / 255.0)
image = tf.image.random_saturation(image, lower=0.5, upper=1.5)
# Make sure the image is still in [0, 1]
image = tf.clip_by_value(image, 0.0, 1.0)
return image, label
batch_size = 32
classes = 13
fileLoc = "C:/Users/USAgData/TF/Clock.csv"
file = pd.read_csv(fileLoc)
file['Loc']=''
file.Loc = str(str(file.Location)[9:23] + str(file.Location)[28:46])
one_hot_Hr = tf.one_hot(file.Hr,classes)
#one_hot_Hr = tf.to_int32(one_hot_Hr)
dataset = tf.data.Dataset.from_tensor_slices((file.Loc, one_hot_Hr))
dataset = dataset.shuffle(len(file.Location))
dataset = dataset.map(parse_function, num_parallel_calls=4)
dataset = dataset.map(train_preprocess, num_parallel_calls=4)
dataset = dataset.batch(batch_size)
dataset = dataset.prefetch(1)
#print(dataset.shape) # ==> "(tf.float32, tf.float32)"
iterator = dataset.make_one_shot_iterator()
next_element = iterator.get_next()
#print(next_element)
tf.keras.backend.clear_session()
model_name="Documentation"
model = k.Sequential()
model.add(Conv2D(64, (3, 3), input_shape=(300,300,3))) #Changed shape to include batch
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
#model.add(Conv2D(32, (3, 3)))
#model.add(Activation('relu'))
#model.add(MaxPooling2D(pool_size=(2, 2)))
#model.add(Conv2D(64, (3, 3)))
#model.add(Activation('relu'))
#model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten())
model.add(Dense(32))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(classes))
model.add(Activation('softmax')) #Changed from sigmoid
#changed from categorical cross entropy
model.compile(loss='categorical_crossentropy',
optimizer=tf.train.RMSPropOptimizer(.0001),
metrics=['accuracy'])
model.summary()
fitting = model.fit_generator(iterator,epochs =1 ,shuffle=False, steps_per_epoch=14400//batch_size)
#model.evaluate(dataset,steps=30)
import sys
print(sys.version)
tf.__version__
I'm running:
tf: 1.10.0
Python: 3.6.7 |Anaconda custom (64-bit)| (default, Dec 10 2018, 20:35:02) [MSC v.1915 64 bit (AMD64)]
I don't know if this should truly be the solution, but when I switch:
fitting = model.fit_generator(iterator,epochs =1 ,shuffle=False, steps_per_epoch=14400//batch_size)
to
fitting = model.fit(iterator,epochs = 1 , shuffle = False, steps_per_epoch = 14400//batch_size)
The model does start to train. But, then them model runs out of data points because the iterator will not start over again.
python tensorflow keras tensorflow-datasets
I'm trying to take data from a csv with a list of files and a list of labels, and convert it to being one-hot labeled for a categorical classification using tf.keras. I am using eager mode for the code.
I'm trying to follow the tf.data example from CS230 building a data pipeline.
https://cs230-stanford.github.io/tensorflow-input-data.html
my code is below under the code section.
the csv file that lists the location of all the pictures is located on dropbox here:
https://www.dropbox.com/s/5uo8o1p30g2aeta/Clock.csv?dl=0
When I run the code as shown below I get a
TypeError: Cannot convert a TensorShape to dtype: <dtype: 'float32'>
error.
When I add to line 55 and make line 56 :
one_hot_Hr = tf.one_hot(file.Hr,classes)
one_hot_Hr = tf.to_int32(one_hot_Hr)
I get this error:
InvalidArgumentError: cannot compute Mul as input #0 was expected to be
a float tensor but is a int32 tensor [Op:Mul]
name: loss/activation_2_loss/mul/
when I run
iterator.get_next()
the pictures are formated as
<tf.Tensor: id=12462, shape=(32, 300, 300, 3), dtype=float32, numpy=
the labels are formated as:
<tf.Tensor: id=12463, shape=(32, 13), dtype=float32, numpy=
based on the errors, it seems like it should be a simple formatting issue with the labels, but I'm stumped and neither error brings up much useful information on stack overflow.
Code:
import pandas as pd
import tensorflow as tf
import tensorflow.keras as k
#import cv2
#tf.enable_eager_execution()
#import argparse
#from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras.layers import Conv2D, MaxPooling2D
from tensorflow.keras.layers import Activation, Dropout, Flatten, Dense
def parse_function(filename, label):
image_string = tf.read_file(filename)
# Don't use tf.image.decode_image, or the output shape will be undefined
image = tf.image.decode_jpeg(image_string, channels=3)
# This will convert to float values in [0, 1]
image = tf.image.convert_image_dtype(image, tf.float32)
image = tf.image.resize_images(image, [300, 300])
return image, label
def train_preprocess(image, label):
image = tf.image.random_flip_left_right(image)
image = tf.image.random_brightness(image, max_delta=32.0 / 255.0)
image = tf.image.random_saturation(image, lower=0.5, upper=1.5)
# Make sure the image is still in [0, 1]
image = tf.clip_by_value(image, 0.0, 1.0)
return image, label
batch_size = 32
classes = 13
fileLoc = "C:/Users/USAgData/TF/Clock.csv"
file = pd.read_csv(fileLoc)
file['Loc']=''
file.Loc = str(str(file.Location)[9:23] + str(file.Location)[28:46])
one_hot_Hr = tf.one_hot(file.Hr,classes)
#one_hot_Hr = tf.to_int32(one_hot_Hr)
dataset = tf.data.Dataset.from_tensor_slices((file.Loc, one_hot_Hr))
dataset = dataset.shuffle(len(file.Location))
dataset = dataset.map(parse_function, num_parallel_calls=4)
dataset = dataset.map(train_preprocess, num_parallel_calls=4)
dataset = dataset.batch(batch_size)
dataset = dataset.prefetch(1)
#print(dataset.shape) # ==> "(tf.float32, tf.float32)"
iterator = dataset.make_one_shot_iterator()
next_element = iterator.get_next()
#print(next_element)
tf.keras.backend.clear_session()
model_name="Documentation"
model = k.Sequential()
model.add(Conv2D(64, (3, 3), input_shape=(300,300,3))) #Changed shape to include batch
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
#model.add(Conv2D(32, (3, 3)))
#model.add(Activation('relu'))
#model.add(MaxPooling2D(pool_size=(2, 2)))
#model.add(Conv2D(64, (3, 3)))
#model.add(Activation('relu'))
#model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten())
model.add(Dense(32))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(classes))
model.add(Activation('softmax')) #Changed from sigmoid
#changed from categorical cross entropy
model.compile(loss='categorical_crossentropy',
optimizer=tf.train.RMSPropOptimizer(.0001),
metrics=['accuracy'])
model.summary()
fitting = model.fit_generator(iterator,epochs =1 ,shuffle=False, steps_per_epoch=14400//batch_size)
#model.evaluate(dataset,steps=30)
import sys
print(sys.version)
tf.__version__
I'm running:
tf: 1.10.0
Python: 3.6.7 |Anaconda custom (64-bit)| (default, Dec 10 2018, 20:35:02) [MSC v.1915 64 bit (AMD64)]
I don't know if this should truly be the solution, but when I switch:
fitting = model.fit_generator(iterator,epochs =1 ,shuffle=False, steps_per_epoch=14400//batch_size)
to
fitting = model.fit(iterator,epochs = 1 , shuffle = False, steps_per_epoch = 14400//batch_size)
The model does start to train. But, then them model runs out of data points because the iterator will not start over again.
python tensorflow keras tensorflow-datasets
python tensorflow keras tensorflow-datasets
edited Jan 24 at 11:05
Steve-0 Dev.
asked Jan 20 at 0:24
Steve-0 Dev.Steve-0 Dev.
187
187
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54272541%2fdata-type-error-for-tf-data-dataset-from-tensor-slices-cannot-convert-a-tenso%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54272541%2fdata-type-error-for-tf-data-dataset-from-tensor-slices-cannot-convert-a-tenso%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ck,7UNcNX VT3lCY,jcpVWArt6Pd,Meu,H VNAfLKt YWTdt1rw,6kzy