How do you do conditional hovering in styled components?
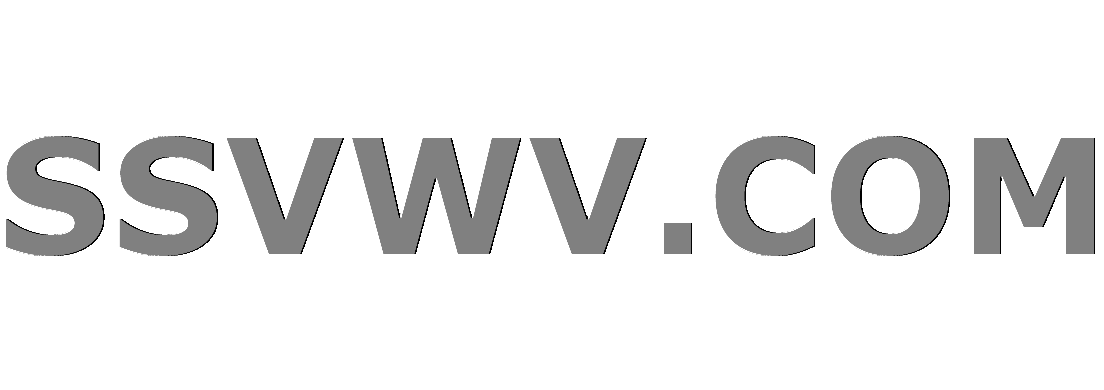
Multi tool use
I have a component that based on certain props I want the ability to change the background on hover. but based on other props, hover should do nothing. is this possible?
export const Container = styled.div`
&:hover {
background: ${({ shouldHover }) => shouldHover ? 'red' : '' };
}
`
however this does not work. any suggestions how this can be done?
javascript reactjs styled-components
add a comment |
I have a component that based on certain props I want the ability to change the background on hover. but based on other props, hover should do nothing. is this possible?
export const Container = styled.div`
&:hover {
background: ${({ shouldHover }) => shouldHover ? 'red' : '' };
}
`
however this does not work. any suggestions how this can be done?
javascript reactjs styled-components
Possible duplicate of Styled Components: props for hover
– AndrewL64
yesterday
add a comment |
I have a component that based on certain props I want the ability to change the background on hover. but based on other props, hover should do nothing. is this possible?
export const Container = styled.div`
&:hover {
background: ${({ shouldHover }) => shouldHover ? 'red' : '' };
}
`
however this does not work. any suggestions how this can be done?
javascript reactjs styled-components
I have a component that based on certain props I want the ability to change the background on hover. but based on other props, hover should do nothing. is this possible?
export const Container = styled.div`
&:hover {
background: ${({ shouldHover }) => shouldHover ? 'red' : '' };
}
`
however this does not work. any suggestions how this can be done?
javascript reactjs styled-components
javascript reactjs styled-components
asked yesterday
Red BaronRed Baron
545
545
Possible duplicate of Styled Components: props for hover
– AndrewL64
yesterday
add a comment |
Possible duplicate of Styled Components: props for hover
– AndrewL64
yesterday
Possible duplicate of Styled Components: props for hover
– AndrewL64
yesterday
Possible duplicate of Styled Components: props for hover
– AndrewL64
yesterday
add a comment |
4 Answers
4
active
oldest
votes
You can try something like the following, that may help:
import { css, styled } from 'styled-components'
styled.div`
${props => props.shouldHover && css`
&:hover {
background: 'red';
}
`}
`
add a comment |
This works for me
import React from "react";
import ReactDOM from "react-dom";
import styled from "styled-components";
const Container = styled.div`
& > h2 {
&:hover {
background: ${props => (props.shouldHover ? "red" : "none")};
}
}
`;
function App({ shouldHover }) {
return (
<Container shouldHover>
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic shappen!</h2>
</Container>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App shouldHover />, rootElement);
Codesandbox
New contributor
tbuglc is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
You can make the background conditional based on what props you pass down. Below is an example where the div changes based on multiple different conditions.
const Container = styled.div`
background: blue;
&:hover {
background: ${props => props.shouldHoverRed
? 'red'
: props.shouldHoverGreen
? 'green'
: props.shouldHoverOrange
? 'orange'
: 'blue'
};
}
`
default is blue and depending on the prop passed it will render different colors for the background on hover.
New contributor
Truitt7 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
This will work:
export const Container = styled.div`
${ props => props.shouldHover
? '&:hover { background: red }'
: ''
}
`;
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54251266%2fhow-do-you-do-conditional-hovering-in-styled-components%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can try something like the following, that may help:
import { css, styled } from 'styled-components'
styled.div`
${props => props.shouldHover && css`
&:hover {
background: 'red';
}
`}
`
add a comment |
You can try something like the following, that may help:
import { css, styled } from 'styled-components'
styled.div`
${props => props.shouldHover && css`
&:hover {
background: 'red';
}
`}
`
add a comment |
You can try something like the following, that may help:
import { css, styled } from 'styled-components'
styled.div`
${props => props.shouldHover && css`
&:hover {
background: 'red';
}
`}
`
You can try something like the following, that may help:
import { css, styled } from 'styled-components'
styled.div`
${props => props.shouldHover && css`
&:hover {
background: 'red';
}
`}
`
answered yesterday


Piyush ZalaniPiyush Zalani
729217
729217
add a comment |
add a comment |
This works for me
import React from "react";
import ReactDOM from "react-dom";
import styled from "styled-components";
const Container = styled.div`
& > h2 {
&:hover {
background: ${props => (props.shouldHover ? "red" : "none")};
}
}
`;
function App({ shouldHover }) {
return (
<Container shouldHover>
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic shappen!</h2>
</Container>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App shouldHover />, rootElement);
Codesandbox
New contributor
tbuglc is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
This works for me
import React from "react";
import ReactDOM from "react-dom";
import styled from "styled-components";
const Container = styled.div`
& > h2 {
&:hover {
background: ${props => (props.shouldHover ? "red" : "none")};
}
}
`;
function App({ shouldHover }) {
return (
<Container shouldHover>
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic shappen!</h2>
</Container>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App shouldHover />, rootElement);
Codesandbox
New contributor
tbuglc is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
This works for me
import React from "react";
import ReactDOM from "react-dom";
import styled from "styled-components";
const Container = styled.div`
& > h2 {
&:hover {
background: ${props => (props.shouldHover ? "red" : "none")};
}
}
`;
function App({ shouldHover }) {
return (
<Container shouldHover>
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic shappen!</h2>
</Container>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App shouldHover />, rootElement);
Codesandbox
New contributor
tbuglc is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
This works for me
import React from "react";
import ReactDOM from "react-dom";
import styled from "styled-components";
const Container = styled.div`
& > h2 {
&:hover {
background: ${props => (props.shouldHover ? "red" : "none")};
}
}
`;
function App({ shouldHover }) {
return (
<Container shouldHover>
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic shappen!</h2>
</Container>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App shouldHover />, rootElement);
Codesandbox
New contributor
tbuglc is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
tbuglc is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered yesterday
tbuglctbuglc
986
986
New contributor
tbuglc is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
tbuglc is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
tbuglc is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
You can make the background conditional based on what props you pass down. Below is an example where the div changes based on multiple different conditions.
const Container = styled.div`
background: blue;
&:hover {
background: ${props => props.shouldHoverRed
? 'red'
: props.shouldHoverGreen
? 'green'
: props.shouldHoverOrange
? 'orange'
: 'blue'
};
}
`
default is blue and depending on the prop passed it will render different colors for the background on hover.
New contributor
Truitt7 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
You can make the background conditional based on what props you pass down. Below is an example where the div changes based on multiple different conditions.
const Container = styled.div`
background: blue;
&:hover {
background: ${props => props.shouldHoverRed
? 'red'
: props.shouldHoverGreen
? 'green'
: props.shouldHoverOrange
? 'orange'
: 'blue'
};
}
`
default is blue and depending on the prop passed it will render different colors for the background on hover.
New contributor
Truitt7 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
You can make the background conditional based on what props you pass down. Below is an example where the div changes based on multiple different conditions.
const Container = styled.div`
background: blue;
&:hover {
background: ${props => props.shouldHoverRed
? 'red'
: props.shouldHoverGreen
? 'green'
: props.shouldHoverOrange
? 'orange'
: 'blue'
};
}
`
default is blue and depending on the prop passed it will render different colors for the background on hover.
New contributor
Truitt7 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
You can make the background conditional based on what props you pass down. Below is an example where the div changes based on multiple different conditions.
const Container = styled.div`
background: blue;
&:hover {
background: ${props => props.shouldHoverRed
? 'red'
: props.shouldHoverGreen
? 'green'
: props.shouldHoverOrange
? 'orange'
: 'blue'
};
}
`
default is blue and depending on the prop passed it will render different colors for the background on hover.
New contributor
Truitt7 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Truitt7 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 22 hours ago


Truitt7Truitt7
1
1
New contributor
Truitt7 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Truitt7 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Truitt7 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
This will work:
export const Container = styled.div`
${ props => props.shouldHover
? '&:hover { background: red }'
: ''
}
`;
add a comment |
This will work:
export const Container = styled.div`
${ props => props.shouldHover
? '&:hover { background: red }'
: ''
}
`;
add a comment |
This will work:
export const Container = styled.div`
${ props => props.shouldHover
? '&:hover { background: red }'
: ''
}
`;
This will work:
export const Container = styled.div`
${ props => props.shouldHover
? '&:hover { background: red }'
: ''
}
`;
edited 21 hours ago
answered yesterday


dmraptisdmraptis
14310
14310
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54251266%2fhow-do-you-do-conditional-hovering-in-styled-components%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CKOOWky,FI2ywB6dpzLhF1MqhpCy vjGbMr0Yeu,dPV34pQCK5e 4JulvL 3,0 5VgC9 sUx 0LnEcaW
Possible duplicate of Styled Components: props for hover
– AndrewL64
yesterday