how to do parsing by quotes and commas subject to quotation marks at the beginning or end?
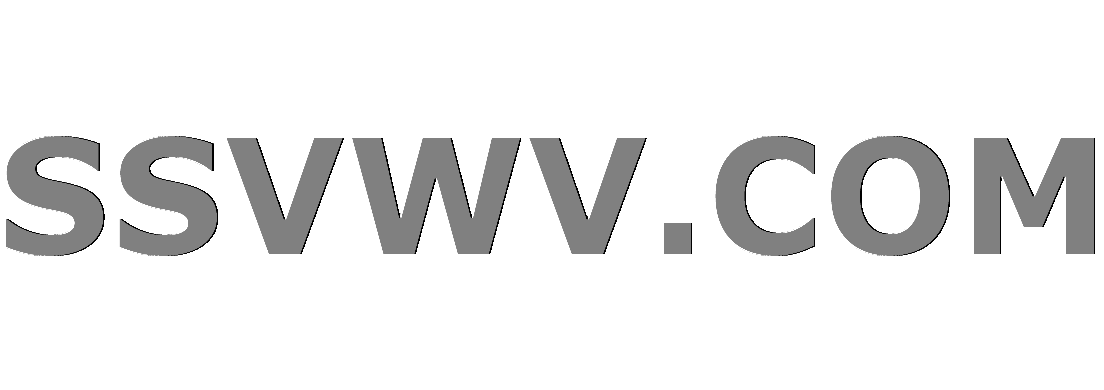
Multi tool use
My program takes a string from the file and divides it into quotes and then into commas, all commas in quotes are part of the text.
static void Main(string args)
{
string source = File.ReadAllLines("file.csv", Encoding.Default);
string src = source[0];
//text preparation for spliting
string modifiedSrc = src.Replace("",", """).Replace(", "", """);
List<string> data = new List<string>();
string parts = modifiedSrc.Split('"');
//odd elements separated by quotes, even by commas
data.AddRange(parts.Where((x, index) => index % 2 != 0));
data.AddRange(parts.Where((x, index) => index % 2 == 0).SelectMany(x => x.Split(',')));
string result = string.Join(" | ", data.Where(x => !string.IsNullOrWhiteSpace(x)));
Console.WriteLine(result);
Console.ReadKey();
}
text: cat, dog, "big, snake", wolf
will show: cat | dog | big, snake | wolf
text: cat, dog, "big, snake", human, "wild, wolf"
will show: cat | dog | big, snake | human | wild, wolf
BUT if there is only one text in quotes and it is not correct at the end or at the beginning:
text: "big, cat", dog, human
will show: big, cat | dog, human
dog, human not shared on: dog | human
How to fix such a special case? I was forbidden to use the library side.
CSV-file https://drive.google.com/file/d/1DtjK6LcI0KLY7B59wD_KkFP3KxZadm5-/view?usp=sharing
c# .net
add a comment |
My program takes a string from the file and divides it into quotes and then into commas, all commas in quotes are part of the text.
static void Main(string args)
{
string source = File.ReadAllLines("file.csv", Encoding.Default);
string src = source[0];
//text preparation for spliting
string modifiedSrc = src.Replace("",", """).Replace(", "", """);
List<string> data = new List<string>();
string parts = modifiedSrc.Split('"');
//odd elements separated by quotes, even by commas
data.AddRange(parts.Where((x, index) => index % 2 != 0));
data.AddRange(parts.Where((x, index) => index % 2 == 0).SelectMany(x => x.Split(',')));
string result = string.Join(" | ", data.Where(x => !string.IsNullOrWhiteSpace(x)));
Console.WriteLine(result);
Console.ReadKey();
}
text: cat, dog, "big, snake", wolf
will show: cat | dog | big, snake | wolf
text: cat, dog, "big, snake", human, "wild, wolf"
will show: cat | dog | big, snake | human | wild, wolf
BUT if there is only one text in quotes and it is not correct at the end or at the beginning:
text: "big, cat", dog, human
will show: big, cat | dog, human
dog, human not shared on: dog | human
How to fix such a special case? I was forbidden to use the library side.
CSV-file https://drive.google.com/file/d/1DtjK6LcI0KLY7B59wD_KkFP3KxZadm5-/view?usp=sharing
c# .net
I ran your code and it runs as expected can you pleas post your csv file. I really feel the error is in the csv file.
– Vedant
Jan 19 at 21:21
CsvHelper.
– Jimi
Jan 19 at 21:40
What happens if there is a space between the comma and the double quote? What happens if there is no space or two spaces?
– jdweng
Jan 19 at 22:18
add a comment |
My program takes a string from the file and divides it into quotes and then into commas, all commas in quotes are part of the text.
static void Main(string args)
{
string source = File.ReadAllLines("file.csv", Encoding.Default);
string src = source[0];
//text preparation for spliting
string modifiedSrc = src.Replace("",", """).Replace(", "", """);
List<string> data = new List<string>();
string parts = modifiedSrc.Split('"');
//odd elements separated by quotes, even by commas
data.AddRange(parts.Where((x, index) => index % 2 != 0));
data.AddRange(parts.Where((x, index) => index % 2 == 0).SelectMany(x => x.Split(',')));
string result = string.Join(" | ", data.Where(x => !string.IsNullOrWhiteSpace(x)));
Console.WriteLine(result);
Console.ReadKey();
}
text: cat, dog, "big, snake", wolf
will show: cat | dog | big, snake | wolf
text: cat, dog, "big, snake", human, "wild, wolf"
will show: cat | dog | big, snake | human | wild, wolf
BUT if there is only one text in quotes and it is not correct at the end or at the beginning:
text: "big, cat", dog, human
will show: big, cat | dog, human
dog, human not shared on: dog | human
How to fix such a special case? I was forbidden to use the library side.
CSV-file https://drive.google.com/file/d/1DtjK6LcI0KLY7B59wD_KkFP3KxZadm5-/view?usp=sharing
c# .net
My program takes a string from the file and divides it into quotes and then into commas, all commas in quotes are part of the text.
static void Main(string args)
{
string source = File.ReadAllLines("file.csv", Encoding.Default);
string src = source[0];
//text preparation for spliting
string modifiedSrc = src.Replace("",", """).Replace(", "", """);
List<string> data = new List<string>();
string parts = modifiedSrc.Split('"');
//odd elements separated by quotes, even by commas
data.AddRange(parts.Where((x, index) => index % 2 != 0));
data.AddRange(parts.Where((x, index) => index % 2 == 0).SelectMany(x => x.Split(',')));
string result = string.Join(" | ", data.Where(x => !string.IsNullOrWhiteSpace(x)));
Console.WriteLine(result);
Console.ReadKey();
}
text: cat, dog, "big, snake", wolf
will show: cat | dog | big, snake | wolf
text: cat, dog, "big, snake", human, "wild, wolf"
will show: cat | dog | big, snake | human | wild, wolf
BUT if there is only one text in quotes and it is not correct at the end or at the beginning:
text: "big, cat", dog, human
will show: big, cat | dog, human
dog, human not shared on: dog | human
How to fix such a special case? I was forbidden to use the library side.
CSV-file https://drive.google.com/file/d/1DtjK6LcI0KLY7B59wD_KkFP3KxZadm5-/view?usp=sharing
c# .net
c# .net
edited Jan 19 at 21:42
van93
asked Jan 19 at 21:07
van93van93
12
12
I ran your code and it runs as expected can you pleas post your csv file. I really feel the error is in the csv file.
– Vedant
Jan 19 at 21:21
CsvHelper.
– Jimi
Jan 19 at 21:40
What happens if there is a space between the comma and the double quote? What happens if there is no space or two spaces?
– jdweng
Jan 19 at 22:18
add a comment |
I ran your code and it runs as expected can you pleas post your csv file. I really feel the error is in the csv file.
– Vedant
Jan 19 at 21:21
CsvHelper.
– Jimi
Jan 19 at 21:40
What happens if there is a space between the comma and the double quote? What happens if there is no space or two spaces?
– jdweng
Jan 19 at 22:18
I ran your code and it runs as expected can you pleas post your csv file. I really feel the error is in the csv file.
– Vedant
Jan 19 at 21:21
I ran your code and it runs as expected can you pleas post your csv file. I really feel the error is in the csv file.
– Vedant
Jan 19 at 21:21
CsvHelper.
– Jimi
Jan 19 at 21:40
CsvHelper.
– Jimi
Jan 19 at 21:40
What happens if there is a space between the comma and the double quote? What happens if there is no space or two spaces?
– jdweng
Jan 19 at 22:18
What happens if there is a space between the comma and the double quote? What happens if there is no space or two spaces?
– jdweng
Jan 19 at 22:18
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54271395%2fhow-to-do-parsing-by-quotes-and-commas-subject-to-quotation-marks-at-the-beginni%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54271395%2fhow-to-do-parsing-by-quotes-and-commas-subject-to-quotation-marks-at-the-beginni%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
m81 kALsNKkhIMHf,O,5 XY,Sfs1bqH1 gJMi7r2r1zAL6yp3qJF2ucn,N
I ran your code and it runs as expected can you pleas post your csv file. I really feel the error is in the csv file.
– Vedant
Jan 19 at 21:21
CsvHelper.
– Jimi
Jan 19 at 21:40
What happens if there is a space between the comma and the double quote? What happens if there is no space or two spaces?
– jdweng
Jan 19 at 22:18