While using the python library rply, I get an unexpected token error when parsing more than one line. How can...
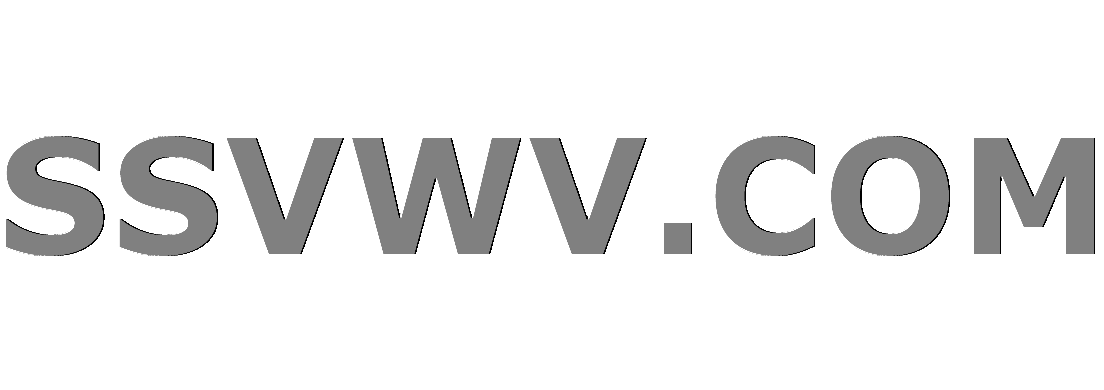
Multi tool use
For the practice, I decided to work on a simple language. When only a single line, my say(); command works fine, but when I do two says in a row, I get an error.
For Parsing I'm using rply. I was following this (https://blog.usejournal.com/writing-your-own-programming-language-and-compiler-with-python-a468970ae6df) guide. I've searched extesively but I cant find a solution.
This is the python code:
from rply import ParserGenerator
from ast import Int, Sum, Sub, Say, String
class Parser():
def __init__(self):
self.pg = ParserGenerator(
# A list of all token names accepted by the parser.
['INTEGER', 'SAY', 'OPEN_PAREN', 'CLOSE_PAREN',
'SEMI_COLON', 'SUM', 'SUB', 'STRING']
)
def parse(self):
@self.pg.production('say : SAY OPEN_PAREN expression CLOSE_PAREN SEMI_COLON')
def say(p):
return Say(p[2])
@self.pg.production('expression : expression SUM expression')
@self.pg.production('expression : expression SUB expression')
def expression(p):
left = p[0]
right = p[2]
operator = p[1]
if operator.gettokentype() == 'SUM':
return Sum(left, right)
elif operator.gettokentype() == 'SUB':
return Sub(left, right)
@self.pg.production('expression : INTEGER')
def int(p):
return Int(p[0].value)
@self.pg.production('expression : STRING')
def string(p):
return String(p[0].value)
@self.pg.error
def error_handler(token):
raise ValueError("Ran into a %s where it wasn't expected" % token.gettokentype())
def get_parser(self):
return self.pg.build()
When I run my program with the input of:
say("yo");
It works fine and return yo.
However, when I input:
say("yo");
say("yoyo");
I expect it to return yo yoyo, but instead I get this error:
C:Usersgdog1Desktopprojintparser.py:42: ParserGeneratorWarning: 4
shift/reduce conflicts
return self.pg.build()
Traceback (most recent call last):
File "<input>", line 1, in <module>
File "C:Program FilesJetBrainsPyCharm Community Edition 2018.3.3helperspydev_pydev_bundlepydev_umd.py", line 197, in runfile
pydev_imports.execfile(filename, global_vars, local_vars) # execute the script
File "C:/Users/gdog1/Desktop/proj/main.py", line 20, in <module>
parser.parse(tokens).eval()
File "C:Python27libsite-packagesrplyparser.py", line 60, in parse
self.error_handler(lookahead)
File "C:Usersgdog1Desktopprojintparser.py", line 39, in error_handler
raise ValueError("Ran into a %s where it wasn't expected" %
token.gettokentype())
ValueError: Ran into a SAY where it wasn't expected
python parsing token
add a comment |
For the practice, I decided to work on a simple language. When only a single line, my say(); command works fine, but when I do two says in a row, I get an error.
For Parsing I'm using rply. I was following this (https://blog.usejournal.com/writing-your-own-programming-language-and-compiler-with-python-a468970ae6df) guide. I've searched extesively but I cant find a solution.
This is the python code:
from rply import ParserGenerator
from ast import Int, Sum, Sub, Say, String
class Parser():
def __init__(self):
self.pg = ParserGenerator(
# A list of all token names accepted by the parser.
['INTEGER', 'SAY', 'OPEN_PAREN', 'CLOSE_PAREN',
'SEMI_COLON', 'SUM', 'SUB', 'STRING']
)
def parse(self):
@self.pg.production('say : SAY OPEN_PAREN expression CLOSE_PAREN SEMI_COLON')
def say(p):
return Say(p[2])
@self.pg.production('expression : expression SUM expression')
@self.pg.production('expression : expression SUB expression')
def expression(p):
left = p[0]
right = p[2]
operator = p[1]
if operator.gettokentype() == 'SUM':
return Sum(left, right)
elif operator.gettokentype() == 'SUB':
return Sub(left, right)
@self.pg.production('expression : INTEGER')
def int(p):
return Int(p[0].value)
@self.pg.production('expression : STRING')
def string(p):
return String(p[0].value)
@self.pg.error
def error_handler(token):
raise ValueError("Ran into a %s where it wasn't expected" % token.gettokentype())
def get_parser(self):
return self.pg.build()
When I run my program with the input of:
say("yo");
It works fine and return yo.
However, when I input:
say("yo");
say("yoyo");
I expect it to return yo yoyo, but instead I get this error:
C:Usersgdog1Desktopprojintparser.py:42: ParserGeneratorWarning: 4
shift/reduce conflicts
return self.pg.build()
Traceback (most recent call last):
File "<input>", line 1, in <module>
File "C:Program FilesJetBrainsPyCharm Community Edition 2018.3.3helperspydev_pydev_bundlepydev_umd.py", line 197, in runfile
pydev_imports.execfile(filename, global_vars, local_vars) # execute the script
File "C:/Users/gdog1/Desktop/proj/main.py", line 20, in <module>
parser.parse(tokens).eval()
File "C:Python27libsite-packagesrplyparser.py", line 60, in parse
self.error_handler(lookahead)
File "C:Usersgdog1Desktopprojintparser.py", line 39, in error_handler
raise ValueError("Ran into a %s where it wasn't expected" %
token.gettokentype())
ValueError: Ran into a SAY where it wasn't expected
python parsing token
add a comment |
For the practice, I decided to work on a simple language. When only a single line, my say(); command works fine, but when I do two says in a row, I get an error.
For Parsing I'm using rply. I was following this (https://blog.usejournal.com/writing-your-own-programming-language-and-compiler-with-python-a468970ae6df) guide. I've searched extesively but I cant find a solution.
This is the python code:
from rply import ParserGenerator
from ast import Int, Sum, Sub, Say, String
class Parser():
def __init__(self):
self.pg = ParserGenerator(
# A list of all token names accepted by the parser.
['INTEGER', 'SAY', 'OPEN_PAREN', 'CLOSE_PAREN',
'SEMI_COLON', 'SUM', 'SUB', 'STRING']
)
def parse(self):
@self.pg.production('say : SAY OPEN_PAREN expression CLOSE_PAREN SEMI_COLON')
def say(p):
return Say(p[2])
@self.pg.production('expression : expression SUM expression')
@self.pg.production('expression : expression SUB expression')
def expression(p):
left = p[0]
right = p[2]
operator = p[1]
if operator.gettokentype() == 'SUM':
return Sum(left, right)
elif operator.gettokentype() == 'SUB':
return Sub(left, right)
@self.pg.production('expression : INTEGER')
def int(p):
return Int(p[0].value)
@self.pg.production('expression : STRING')
def string(p):
return String(p[0].value)
@self.pg.error
def error_handler(token):
raise ValueError("Ran into a %s where it wasn't expected" % token.gettokentype())
def get_parser(self):
return self.pg.build()
When I run my program with the input of:
say("yo");
It works fine and return yo.
However, when I input:
say("yo");
say("yoyo");
I expect it to return yo yoyo, but instead I get this error:
C:Usersgdog1Desktopprojintparser.py:42: ParserGeneratorWarning: 4
shift/reduce conflicts
return self.pg.build()
Traceback (most recent call last):
File "<input>", line 1, in <module>
File "C:Program FilesJetBrainsPyCharm Community Edition 2018.3.3helperspydev_pydev_bundlepydev_umd.py", line 197, in runfile
pydev_imports.execfile(filename, global_vars, local_vars) # execute the script
File "C:/Users/gdog1/Desktop/proj/main.py", line 20, in <module>
parser.parse(tokens).eval()
File "C:Python27libsite-packagesrplyparser.py", line 60, in parse
self.error_handler(lookahead)
File "C:Usersgdog1Desktopprojintparser.py", line 39, in error_handler
raise ValueError("Ran into a %s where it wasn't expected" %
token.gettokentype())
ValueError: Ran into a SAY where it wasn't expected
python parsing token
For the practice, I decided to work on a simple language. When only a single line, my say(); command works fine, but when I do two says in a row, I get an error.
For Parsing I'm using rply. I was following this (https://blog.usejournal.com/writing-your-own-programming-language-and-compiler-with-python-a468970ae6df) guide. I've searched extesively but I cant find a solution.
This is the python code:
from rply import ParserGenerator
from ast import Int, Sum, Sub, Say, String
class Parser():
def __init__(self):
self.pg = ParserGenerator(
# A list of all token names accepted by the parser.
['INTEGER', 'SAY', 'OPEN_PAREN', 'CLOSE_PAREN',
'SEMI_COLON', 'SUM', 'SUB', 'STRING']
)
def parse(self):
@self.pg.production('say : SAY OPEN_PAREN expression CLOSE_PAREN SEMI_COLON')
def say(p):
return Say(p[2])
@self.pg.production('expression : expression SUM expression')
@self.pg.production('expression : expression SUB expression')
def expression(p):
left = p[0]
right = p[2]
operator = p[1]
if operator.gettokentype() == 'SUM':
return Sum(left, right)
elif operator.gettokentype() == 'SUB':
return Sub(left, right)
@self.pg.production('expression : INTEGER')
def int(p):
return Int(p[0].value)
@self.pg.production('expression : STRING')
def string(p):
return String(p[0].value)
@self.pg.error
def error_handler(token):
raise ValueError("Ran into a %s where it wasn't expected" % token.gettokentype())
def get_parser(self):
return self.pg.build()
When I run my program with the input of:
say("yo");
It works fine and return yo.
However, when I input:
say("yo");
say("yoyo");
I expect it to return yo yoyo, but instead I get this error:
C:Usersgdog1Desktopprojintparser.py:42: ParserGeneratorWarning: 4
shift/reduce conflicts
return self.pg.build()
Traceback (most recent call last):
File "<input>", line 1, in <module>
File "C:Program FilesJetBrainsPyCharm Community Edition 2018.3.3helperspydev_pydev_bundlepydev_umd.py", line 197, in runfile
pydev_imports.execfile(filename, global_vars, local_vars) # execute the script
File "C:/Users/gdog1/Desktop/proj/main.py", line 20, in <module>
parser.parse(tokens).eval()
File "C:Python27libsite-packagesrplyparser.py", line 60, in parse
self.error_handler(lookahead)
File "C:Usersgdog1Desktopprojintparser.py", line 39, in error_handler
raise ValueError("Ran into a %s where it wasn't expected" %
token.gettokentype())
ValueError: Ran into a SAY where it wasn't expected
python parsing token
python parsing token
asked Jan 19 at 9:21


George WalkerGeorge Walker
1
1
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Your grammar describes a single command:
say : SAY OPEN_PAREN expression CLOSE_PAREN SEMI_COLON
So that is what the parser accepts.
If you want the input to consist of multiple commands, you need to write a grammar which describes that input:
program :
program : program say
Thanks, that makes sense when I think about it.
– George Walker
Jan 20 at 6:10
add a comment |
As the error, says its with the below line:
raise ValueError("Ran into a %s where it wasn't expected" % token.gettokentype())
Change it as below and check:
raise ValueError('Ran into a %s where it wasn't expected' % (token.gettokentype()))
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54265650%2fwhile-using-the-python-library-rply-i-get-an-unexpected-token-error-when-parsin%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your grammar describes a single command:
say : SAY OPEN_PAREN expression CLOSE_PAREN SEMI_COLON
So that is what the parser accepts.
If you want the input to consist of multiple commands, you need to write a grammar which describes that input:
program :
program : program say
Thanks, that makes sense when I think about it.
– George Walker
Jan 20 at 6:10
add a comment |
Your grammar describes a single command:
say : SAY OPEN_PAREN expression CLOSE_PAREN SEMI_COLON
So that is what the parser accepts.
If you want the input to consist of multiple commands, you need to write a grammar which describes that input:
program :
program : program say
Thanks, that makes sense when I think about it.
– George Walker
Jan 20 at 6:10
add a comment |
Your grammar describes a single command:
say : SAY OPEN_PAREN expression CLOSE_PAREN SEMI_COLON
So that is what the parser accepts.
If you want the input to consist of multiple commands, you need to write a grammar which describes that input:
program :
program : program say
Your grammar describes a single command:
say : SAY OPEN_PAREN expression CLOSE_PAREN SEMI_COLON
So that is what the parser accepts.
If you want the input to consist of multiple commands, you need to write a grammar which describes that input:
program :
program : program say
answered Jan 19 at 12:45
ricirici
153k19135200
153k19135200
Thanks, that makes sense when I think about it.
– George Walker
Jan 20 at 6:10
add a comment |
Thanks, that makes sense when I think about it.
– George Walker
Jan 20 at 6:10
Thanks, that makes sense when I think about it.
– George Walker
Jan 20 at 6:10
Thanks, that makes sense when I think about it.
– George Walker
Jan 20 at 6:10
add a comment |
As the error, says its with the below line:
raise ValueError("Ran into a %s where it wasn't expected" % token.gettokentype())
Change it as below and check:
raise ValueError('Ran into a %s where it wasn't expected' % (token.gettokentype()))
add a comment |
As the error, says its with the below line:
raise ValueError("Ran into a %s where it wasn't expected" % token.gettokentype())
Change it as below and check:
raise ValueError('Ran into a %s where it wasn't expected' % (token.gettokentype()))
add a comment |
As the error, says its with the below line:
raise ValueError("Ran into a %s where it wasn't expected" % token.gettokentype())
Change it as below and check:
raise ValueError('Ran into a %s where it wasn't expected' % (token.gettokentype()))
As the error, says its with the below line:
raise ValueError("Ran into a %s where it wasn't expected" % token.gettokentype())
Change it as below and check:
raise ValueError('Ran into a %s where it wasn't expected' % (token.gettokentype()))
answered Jan 19 at 9:42


Jim ToddJim Todd
42737
42737
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54265650%2fwhile-using-the-python-library-rply-i-get-an-unexpected-token-error-when-parsin%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
a YMK3gRfk yuD cpU9tIlc h6,y2Z2 mCt